mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
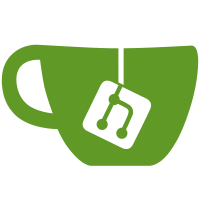
* v3 import renamings * tidy * fmt * rev * Update beacon-chain/core/epoch/precompute/reward_penalty_test.go * Update beacon-chain/core/helpers/validators_test.go * Update beacon-chain/db/alias.go * Update beacon-chain/db/alias.go * Update beacon-chain/db/alias.go * Update beacon-chain/db/iface/BUILD.bazel * Update beacon-chain/db/kv/kv.go * Update beacon-chain/db/kv/state.go * Update beacon-chain/rpc/prysm/v1alpha1/validator/attester_test.go * Update beacon-chain/rpc/prysm/v1alpha1/validator/attester_test.go * Update beacon-chain/sync/initial-sync/service.go * fix deps * fix bad replacements * fix bad replacements * change back * gohashtree version * fix deps Co-authored-by: Nishant Das <nishdas93@gmail.com> Co-authored-by: Potuz <potuz@prysmaticlabs.com>
44 lines
1.5 KiB
Go
44 lines
1.5 KiB
Go
package slasher
|
|
|
|
import (
|
|
"context"
|
|
|
|
types "github.com/prysmaticlabs/prysm/v3/consensus-types/primitives"
|
|
ethpb "github.com/prysmaticlabs/prysm/v3/proto/prysm/v1alpha1"
|
|
"google.golang.org/grpc/codes"
|
|
"google.golang.org/grpc/status"
|
|
)
|
|
|
|
// IsSlashableAttestation returns an attester slashing if an input
|
|
// attestation is found to be slashable.
|
|
func (s *Server) IsSlashableAttestation(
|
|
ctx context.Context, req *ethpb.IndexedAttestation,
|
|
) (*ethpb.AttesterSlashingResponse, error) {
|
|
attesterSlashings, err := s.SlashingChecker.IsSlashableAttestation(ctx, req)
|
|
if err != nil {
|
|
return nil, status.Errorf(codes.Internal, "Could not determine if attestation is slashable: %v", err)
|
|
}
|
|
if len(attesterSlashings) > 0 {
|
|
return ðpb.AttesterSlashingResponse{
|
|
AttesterSlashings: attesterSlashings,
|
|
}, nil
|
|
}
|
|
return ðpb.AttesterSlashingResponse{}, nil
|
|
}
|
|
|
|
// HighestAttestations returns the highest source and target epochs attested for
|
|
// validator indices that have been observed by slasher.
|
|
func (s *Server) HighestAttestations(
|
|
ctx context.Context, req *ethpb.HighestAttestationRequest,
|
|
) (*ethpb.HighestAttestationResponse, error) {
|
|
valIndices := make([]types.ValidatorIndex, len(req.ValidatorIndices))
|
|
for i, valIdx := range req.ValidatorIndices {
|
|
valIndices[i] = types.ValidatorIndex(valIdx)
|
|
}
|
|
atts, err := s.SlashingChecker.HighestAttestations(ctx, valIndices)
|
|
if err != nil {
|
|
return nil, status.Errorf(codes.Internal, "Could not get highest attestations: %v", err)
|
|
}
|
|
return ðpb.HighestAttestationResponse{Attestations: atts}, nil
|
|
}
|