mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-26 05:17:22 +00:00
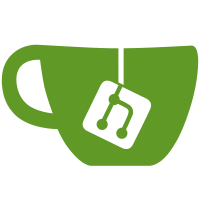
* first version - broken * working proto changes * resolve review remarks * fix goimport issues * fix service issues * first logic version-broken * first running version - no new tests * fix validator client test * add wait group to goroutines * remove unused var in function call * fix review remarks and tests * merge master changes and fix conflicts * gazzele fix * fix prestonvanloon requested changes * merge and some of terenc3t remarks addressed * _,pk bug fix in log * fix account file name suffix and filter not active validator out * merge with master and fix missing parameters * run over all public keys in hasvalidators * add test for error when no all the validators has index in the db and hasvalidators is called * fix runner tests fail due to timing issues * goimports * smaller sleep time in proposer tests * fix UpdateAssignments loging * fix goimports * added && false commented TestUpdateAssignments_DoesNothingWhenNotEpochStartAndAlreadyExistingAssignments * hasvalidators without missing publickeys list * fix some of prestone review remarks * fixes for prestone comments * review changes applied * expect context call in TestWaitForActivation_ValidatorOriginallyExists * changed hasvalidators to return true if one validator exists * fix init problem to getkeys * hasvalidators requiers all validators to be in db * validator attest assignments update * fix ap var name * Change name to hasallvalidators * fix tests * update script, fix any vs all validator calls * fix wait for activation * filter validator * reuse the reply block * fix imports * Remove dup * better lookup of active validators * better filter active vlaidators, still need to fix committee assignment tests * lint * use activated keys * fix for postchainstart * fix logging * move state transitions * hasanyvalidator and hasallvalidators * fix tests with updatechainhead missing * add tests * fix TestCommitteeAssignment_OK * fix test * fix validator tests * fix TestCommitteeAssignment_multipleKeys_OK and TestWaitForActivation_ValidatorOriginallyExists * fix goimports * removed unused param from assignment * change string(pk) to hex.EncodeString(pk) fix change requests * add inactive validator status to assignments * fix logging mess due to multi validator setup * set no assignment to debug level * log assignments every epoch * logging fixes * fixed runtime by using the right assignments * correct activation request * fix the validator panic * correct assignment * fix test fail and waitforactivation * performance log issue fix * fix goimports * add log message with truncated pk for attest * add truncated pk to attest and propose logs * Add comment to script, change 9 to 8 * Update assignment log * Add comment, report number of assignments * Use WithError, add validator as field, merge block proposal log * Update validator_propose.go * fix * use entry.String() * fix fmt
78 lines
2.7 KiB
Go
78 lines
2.7 KiB
Go
package client
|
|
|
|
import (
|
|
"context"
|
|
"encoding/hex"
|
|
"fmt"
|
|
"strings"
|
|
|
|
pb "github.com/prysmaticlabs/prysm/proto/beacon/rpc/v1"
|
|
"github.com/prysmaticlabs/prysm/shared/params"
|
|
"github.com/sirupsen/logrus"
|
|
)
|
|
|
|
// LogValidatorGainsAndLosses logs important metrics related to this validator client's
|
|
// responsibilities throughout the beacon chain's lifecycle. It logs absolute accrued rewards
|
|
// and penalties over time, percentage gain/loss, and gives the end user a better idea
|
|
// of how the validator performs with respect to the rest.
|
|
func (v *validator) LogValidatorGainsAndLosses(ctx context.Context, slot uint64) error {
|
|
if slot%params.BeaconConfig().SlotsPerEpoch != 0 {
|
|
// Do nothing if we are not at the start of a new epoch.
|
|
return nil
|
|
}
|
|
epoch := slot / params.BeaconConfig().SlotsPerEpoch
|
|
if epoch == params.BeaconConfig().GenesisEpoch {
|
|
v.prevBalance = params.BeaconConfig().MaxDepositAmount
|
|
}
|
|
var totalPrevBalance uint64
|
|
reported := false
|
|
for _, pkey := range v.pubkeys {
|
|
req := &pb.ValidatorPerformanceRequest{
|
|
Slot: slot,
|
|
PublicKey: pkey,
|
|
}
|
|
resp, err := v.validatorClient.ValidatorPerformance(ctx, req)
|
|
if err != nil {
|
|
if strings.Contains(err.Error(), "could not get validator index") {
|
|
continue
|
|
}
|
|
return err
|
|
}
|
|
tpk := hex.EncodeToString(pkey)[:12]
|
|
if !reported {
|
|
log.WithFields(logrus.Fields{
|
|
"slot": slot - params.BeaconConfig().GenesisSlot,
|
|
"epoch": (slot / params.BeaconConfig().SlotsPerEpoch) - params.BeaconConfig().GenesisEpoch,
|
|
}).Info("Start of a new epoch!")
|
|
log.WithFields(logrus.Fields{
|
|
"totalValidators": resp.TotalValidators,
|
|
"numActiveValidators": resp.TotalActiveValidators,
|
|
}).Infof("Validator registry information")
|
|
log.Info("Generating validator performance report from the previous epoch...")
|
|
avgBalance := resp.AverageValidatorBalance / float32(params.BeaconConfig().GweiPerEth)
|
|
log.WithField(
|
|
"averageEthBalance", fmt.Sprintf("%f", avgBalance),
|
|
).Info("Average eth balance per validator in the beacon chain")
|
|
reported = true
|
|
}
|
|
newBalance := float64(resp.Balance) / float64(params.BeaconConfig().GweiPerEth)
|
|
log.WithFields(logrus.Fields{
|
|
"ethBalance": newBalance,
|
|
}).Infof("%v New validator balance", tpk)
|
|
|
|
if v.prevBalance > 0 {
|
|
prevBalance := float64(v.prevBalance) / float64(params.BeaconConfig().GweiPerEth)
|
|
percentNet := (newBalance - prevBalance) / prevBalance
|
|
log.WithField("prevEthBalance", prevBalance).Infof("%v Previous validator balance", tpk)
|
|
log.WithFields(logrus.Fields{
|
|
"eth": fmt.Sprintf("%f", newBalance-prevBalance),
|
|
"percentChange": fmt.Sprintf("%.2f%%", percentNet*100),
|
|
}).Infof("%v Net gains/losses in eth", tpk)
|
|
}
|
|
totalPrevBalance += resp.Balance
|
|
}
|
|
|
|
v.prevBalance = totalPrevBalance
|
|
return nil
|
|
}
|