mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
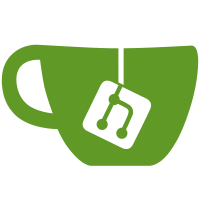
* v3 import renamings * tidy * fmt * rev * Update beacon-chain/core/epoch/precompute/reward_penalty_test.go * Update beacon-chain/core/helpers/validators_test.go * Update beacon-chain/db/alias.go * Update beacon-chain/db/alias.go * Update beacon-chain/db/alias.go * Update beacon-chain/db/iface/BUILD.bazel * Update beacon-chain/db/kv/kv.go * Update beacon-chain/db/kv/state.go * Update beacon-chain/rpc/prysm/v1alpha1/validator/attester_test.go * Update beacon-chain/rpc/prysm/v1alpha1/validator/attester_test.go * Update beacon-chain/sync/initial-sync/service.go * fix deps * fix bad replacements * fix bad replacements * change back * gohashtree version * fix deps Co-authored-by: Nishant Das <nishdas93@gmail.com> Co-authored-by: Potuz <potuz@prysmaticlabs.com>
37 lines
1.3 KiB
Go
37 lines
1.3 KiB
Go
package helpers
|
|
|
|
import (
|
|
"errors"
|
|
|
|
"github.com/prysmaticlabs/prysm/v3/beacon-chain/rpc/statefetcher"
|
|
"github.com/prysmaticlabs/prysm/v3/beacon-chain/state/stategen"
|
|
"google.golang.org/grpc/codes"
|
|
"google.golang.org/grpc/status"
|
|
)
|
|
|
|
// PrepareStateFetchGRPCError returns an appropriate gRPC error based on the supplied argument.
|
|
// The argument error should be a result of fetching state.
|
|
func PrepareStateFetchGRPCError(err error) error {
|
|
if errors.Is(err, stategen.ErrNoDataForSlot) {
|
|
return status.Errorf(codes.NotFound, "lacking historical data needed to fulfill request")
|
|
}
|
|
if stateNotFoundErr, ok := err.(*statefetcher.StateNotFoundError); ok {
|
|
return status.Errorf(codes.NotFound, "State not found: %v", stateNotFoundErr)
|
|
}
|
|
if parseErr, ok := err.(*statefetcher.StateIdParseError); ok {
|
|
return status.Errorf(codes.InvalidArgument, "Invalid state ID: %v", parseErr)
|
|
}
|
|
return status.Errorf(codes.Internal, "Invalid state ID: %v", err)
|
|
}
|
|
|
|
// IndexedVerificationFailure represents a collection of verification failures.
|
|
type IndexedVerificationFailure struct {
|
|
Failures []*SingleIndexedVerificationFailure `json:"failures"`
|
|
}
|
|
|
|
// SingleIndexedVerificationFailure represents an issue when verifying a single indexed object e.g. an item in an array.
|
|
type SingleIndexedVerificationFailure struct {
|
|
Index int `json:"index"`
|
|
Message string `json:"message"`
|
|
}
|