mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-23 20:07:17 +00:00
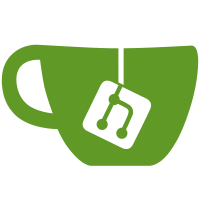
* WIP * Refactor to use iface.ValidatorClient instead of ethpb.BeaconNodeValidatorClient * Add mocks for iface.ValidatorClient * Fix mocks * Update update-mockgen.sh * Fix warnings * Fix config_setting syntax * Use custom build settings * WIP * WIP * WIP * WIP * WIP * WIP * Fix endpoint address and reduce timeout * Revert most e2e changes * Use e2e.TestParams.Ports.PrysmBeaconNodeGatewayPort * Fix BeaconRESTApiProviderFlag port * Revert e2e changes
44 lines
1015 B
Go
44 lines
1015 B
Go
package validator_helpers
|
|
|
|
import (
|
|
"time"
|
|
|
|
"google.golang.org/grpc"
|
|
)
|
|
|
|
// Use an interface with a private dummy function to force all other packages to call NewNodeConnection
|
|
type NodeConnection interface {
|
|
GetGrpcClientConn() *grpc.ClientConn
|
|
GetBeaconApiUrl() string
|
|
GetBeaconApiTimeout() time.Duration
|
|
dummy()
|
|
}
|
|
|
|
type nodeConnection struct {
|
|
grpcClientConn *grpc.ClientConn
|
|
beaconApiUrl string
|
|
beaconApiTimeout time.Duration
|
|
}
|
|
|
|
func (c *nodeConnection) GetGrpcClientConn() *grpc.ClientConn {
|
|
return c.grpcClientConn
|
|
}
|
|
|
|
func (c *nodeConnection) GetBeaconApiUrl() string {
|
|
return c.beaconApiUrl
|
|
}
|
|
|
|
func (c *nodeConnection) GetBeaconApiTimeout() time.Duration {
|
|
return c.beaconApiTimeout
|
|
}
|
|
|
|
func (*nodeConnection) dummy() {}
|
|
|
|
func NewNodeConnection(grpcConn *grpc.ClientConn, beaconApiUrl string, beaconApiTimeout time.Duration) NodeConnection {
|
|
conn := &nodeConnection{}
|
|
conn.grpcClientConn = grpcConn
|
|
conn.beaconApiUrl = beaconApiUrl
|
|
conn.beaconApiTimeout = beaconApiTimeout
|
|
return conn
|
|
}
|