mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 04:47:18 +00:00
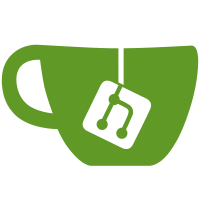
* add lock analyzer * fix locks * progress * fix failures * fix error log Co-authored-by: Raul Jordan <raul@prysmaticlabs.com>
45 lines
1.1 KiB
Go
45 lines
1.1 KiB
Go
// These nested rlock patterns are too complex for the analyzer to catch right now
|
|
package testdata
|
|
|
|
func (p *ProtectResource) FuncLitInStructLit() {
|
|
p.RLock()
|
|
type funcLitContainer struct {
|
|
funcLit func()
|
|
}
|
|
var fl *funcLitContainer = &funcLitContainer{
|
|
funcLit: func() {
|
|
p.RLock()
|
|
},
|
|
}
|
|
fl.funcLit() // this is a nested RLock but won't be caught
|
|
p.RUnlock()
|
|
}
|
|
|
|
func (p *ProtectResource) FuncLitInStructLitLocked() {
|
|
p.Lock()
|
|
type funcLitContainer struct {
|
|
funcLit func()
|
|
}
|
|
var fl *funcLitContainer = &funcLitContainer{
|
|
funcLit: func() {
|
|
p.Lock()
|
|
},
|
|
}
|
|
fl.funcLit() // this is a nested Lock but won't be caught
|
|
p.Unlock()
|
|
}
|
|
|
|
func (e *ExposedMutex) FuncReturnsMutex() {
|
|
e.GetLock().RLock()
|
|
e.lock.RLock() // this is an obvious nested lock, but won't be caught since the first RLock was called through a getter function
|
|
e.lock.RUnlock()
|
|
e.GetLock().RUnlock()
|
|
}
|
|
|
|
func (e *ExposedMutex) FuncReturnsMutexLocked() {
|
|
e.GetLock().Lock()
|
|
e.lock.Lock() // this is an obvious nested lock, but won't be caught since the first RLock was called through a getter function
|
|
e.lock.Unlock()
|
|
e.GetLock().Unlock()
|
|
}
|