mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-26 05:17:22 +00:00
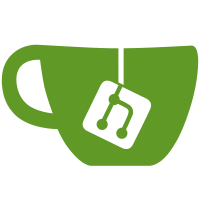
* db methods: remove Get prefix for getter functions * db methods: consistent test names by removing Get
44 lines
1.1 KiB
Go
44 lines
1.1 KiB
Go
package db
|
|
|
|
import (
|
|
"errors"
|
|
|
|
"github.com/boltdb/bolt"
|
|
)
|
|
|
|
// CleanedFinalizedSlot returns the most recent finalized slot when we did a DB clean up.
|
|
func (db *BeaconDB) CleanedFinalizedSlot() (uint64, error) {
|
|
var lastFinalizedSlot uint64
|
|
|
|
err := db.view(func(tx *bolt.Tx) error {
|
|
cleanupHistory := tx.Bucket(cleanupHistoryBucket)
|
|
|
|
slotEnc := cleanupHistory.Get(cleanedFinalizedSlotKey)
|
|
// If last cleaned slot number is not found, we will return 0 instead
|
|
if slotEnc == nil {
|
|
return nil
|
|
}
|
|
|
|
lastFinalizedSlot = decodeToSlotNumber(slotEnc)
|
|
return nil
|
|
})
|
|
|
|
return lastFinalizedSlot, err
|
|
}
|
|
|
|
// SaveCleanedFinalizedSlot writes the slot when we did DB cleanup so we can start from here in future cleanup tasks.
|
|
func (db *BeaconDB) SaveCleanedFinalizedSlot(slot uint64) error {
|
|
slotEnc := encodeSlotNumber(slot)
|
|
|
|
err := db.update(func(tx *bolt.Tx) error {
|
|
cleanupHistory := tx.Bucket(cleanupHistoryBucket)
|
|
|
|
if err := cleanupHistory.Put(cleanedFinalizedSlotKey, slotEnc); err != nil {
|
|
return errors.New("failed to store cleaned finalized slot in DB")
|
|
}
|
|
|
|
return nil
|
|
})
|
|
return err
|
|
}
|