mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 12:57:18 +00:00
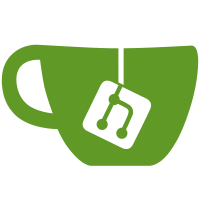
* Add REST implementation for Validator's PrepareBeaconProposer * Change fee recipients * Handle error in a better way * Move endpoint into constant Co-authored-by: Radosław Kapka <rkapka@wp.pl>
34 lines
1.1 KiB
Go
34 lines
1.1 KiB
Go
package beacon_api
|
|
|
|
import (
|
|
"bytes"
|
|
"encoding/json"
|
|
"strconv"
|
|
|
|
"github.com/ethereum/go-ethereum/common/hexutil"
|
|
"github.com/pkg/errors"
|
|
"github.com/prysmaticlabs/prysm/v3/beacon-chain/rpc/apimiddleware"
|
|
ethpb "github.com/prysmaticlabs/prysm/v3/proto/prysm/v1alpha1"
|
|
)
|
|
|
|
func (c *beaconApiValidatorClient) prepareBeaconProposer(recipients []*ethpb.PrepareBeaconProposerRequest_FeeRecipientContainer) error {
|
|
jsonRecipients := make([]*apimiddleware.FeeRecipientJson, len(recipients))
|
|
for index, recipient := range recipients {
|
|
jsonRecipients[index] = &apimiddleware.FeeRecipientJson{
|
|
FeeRecipient: hexutil.Encode(recipient.FeeRecipient),
|
|
ValidatorIndex: strconv.FormatUint(uint64(recipient.ValidatorIndex), 10),
|
|
}
|
|
}
|
|
|
|
marshalledJsonRecipients, err := json.Marshal(jsonRecipients)
|
|
if err != nil {
|
|
return errors.Wrap(err, "failed to marshal recipients")
|
|
}
|
|
|
|
if _, err := c.jsonRestHandler.PostRestJson("/eth/v1/validator/prepare_beacon_proposer", nil, bytes.NewBuffer(marshalledJsonRecipients), nil); err != nil {
|
|
return errors.Wrap(err, "failed to send POST data to REST endpoint")
|
|
}
|
|
|
|
return nil
|
|
}
|