mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-26 05:17:22 +00:00
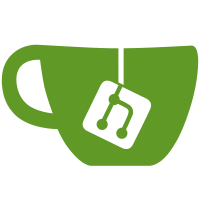
* WIP: Dummy db clean service * [WIP. NOT READY FOR REVIEW] Add DB cleanup routine that cleans block vote cache * Add missing bazel config * Put DB clean behind a CLI flag * Address review comments * Fix error handling
62 lines
1.8 KiB
Go
62 lines
1.8 KiB
Go
package db
|
|
|
|
import (
|
|
"fmt"
|
|
|
|
"github.com/boltdb/bolt"
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/utils"
|
|
)
|
|
|
|
// ReadBlockVoteCache read block vote cache from DB.
|
|
func (db *BeaconDB) ReadBlockVoteCache(blockHashes [][32]byte) (utils.BlockVoteCache, error) {
|
|
blockVoteCache := utils.NewBlockVoteCache()
|
|
err := db.view(func(tx *bolt.Tx) error {
|
|
blockVoteCacheInfo := tx.Bucket(blockVoteCacheBucket)
|
|
for _, h := range blockHashes {
|
|
blob := blockVoteCacheInfo.Get(h[:])
|
|
if blob == nil {
|
|
continue
|
|
}
|
|
vote := new(utils.BlockVote)
|
|
if err := vote.Unmarshal(blob); err != nil {
|
|
return fmt.Errorf("failed to decode block vote cache for block hash %x", h)
|
|
}
|
|
blockVoteCache[h] = vote
|
|
}
|
|
return nil
|
|
})
|
|
return blockVoteCache, err
|
|
}
|
|
|
|
// DeleteBlockVoteCache removes vote cache for specified blocks from DB.
|
|
func (db *BeaconDB) DeleteBlockVoteCache(blockHashes [][32]byte) error {
|
|
err := db.update(func(tx *bolt.Tx) error {
|
|
blockVoteCacheInfo := tx.Bucket(blockVoteCacheBucket)
|
|
for _, h := range blockHashes {
|
|
if err := blockVoteCacheInfo.Delete(h[:]); err != nil {
|
|
return fmt.Errorf("failed to delete block vote cache for block hash %x: %v", h, err)
|
|
}
|
|
}
|
|
return nil
|
|
})
|
|
return err
|
|
}
|
|
|
|
// WriteBlockVoteCache write block vote cache object into DB.
|
|
func (db *BeaconDB) WriteBlockVoteCache(blockVoteCache utils.BlockVoteCache) error {
|
|
err := db.update(func(tx *bolt.Tx) error {
|
|
blockVoteCacheInfo := tx.Bucket(blockVoteCacheBucket)
|
|
for h := range blockVoteCache {
|
|
blob, err := blockVoteCache[h].Marshal()
|
|
if err != nil {
|
|
return fmt.Errorf("failed to encode block vote cache for block hash %x", h)
|
|
}
|
|
if err = blockVoteCacheInfo.Put(h[:], blob); err != nil {
|
|
return fmt.Errorf("failed to store block vote cache into DB")
|
|
}
|
|
}
|
|
return nil
|
|
})
|
|
return err
|
|
}
|