mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-26 21:27:19 +00:00
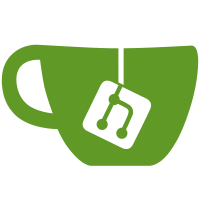
* plug forkchoice to blockchain service's block processing * fixed tests * more fixes... * clean ups * fixed test * Update beacon-chain/blockchain/block_processing.go * merged with 2006 and started fixing tests * remove prints * fixed tests * lint * include ops service * if there's a skip slot, slot-- * fixed typo * started working on test * no fork choice in propose * bleh, need to fix state generator first * state gen takes input slot * feedback * fixed tests * preston's feedback * fmt * removed extra logging * add more logs * fixed validator attest * builds * fixed save block * children fix * removed verbose logs * fix fork choice * right logs * Add Prometheus Counter for Reorg (#2051) * fetch every slot (#2052) * test Fixes * lint * only regenerate state if there was a reorg * better logging * fixed seed * better logging * process skip slots in assignment requests * fix lint * disable state root computation * filter attestations in regular sync * log important items * better info logs * added spans to stategen * span in stategen * set validator deadline * randao stuff * disable sig verify * lint * lint * save only using historical states * use new goroutine for handling sync messages * change default buffer sizes * better p2p * rem some useless logs * lint * sync tests complete * complete tests * tests fixed * lint * fix flakey att service * PR feedback * undo k8s changes * Update beacon-chain/blockchain/block_processing.go * Update beacon-chain/sync/regular_sync.go * Add feature flag to enable compute state root * add comment * gazelle lint fix
118 lines
2.8 KiB
Go
118 lines
2.8 KiB
Go
package sync
|
|
|
|
import (
|
|
"context"
|
|
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/db"
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/operations"
|
|
initialsync "github.com/prysmaticlabs/prysm/beacon-chain/sync/initial-sync"
|
|
"github.com/sirupsen/logrus"
|
|
)
|
|
|
|
var slog = logrus.WithField("prefix", "sync")
|
|
|
|
// Service defines the main routines used in the sync service.
|
|
type Service struct {
|
|
RegularSync *RegularSync
|
|
InitialSync *initialsync.InitialSync
|
|
Querier *Querier
|
|
}
|
|
|
|
// Config defines the configured services required for sync to work.
|
|
type Config struct {
|
|
ChainService chainService
|
|
BeaconDB *db.BeaconDB
|
|
P2P p2pAPI
|
|
AttsService attsService
|
|
OperationService operations.OperationFeeds
|
|
PowChainService powChainService
|
|
}
|
|
|
|
// NewSyncService creates a new instance of SyncService using the config
|
|
// given.
|
|
func NewSyncService(ctx context.Context, cfg *Config) *Service {
|
|
|
|
sqCfg := DefaultQuerierConfig()
|
|
sqCfg.BeaconDB = cfg.BeaconDB
|
|
sqCfg.P2P = cfg.P2P
|
|
sqCfg.PowChain = cfg.PowChainService
|
|
sqCfg.ChainService = cfg.ChainService
|
|
|
|
isCfg := initialsync.DefaultConfig()
|
|
isCfg.BeaconDB = cfg.BeaconDB
|
|
isCfg.P2P = cfg.P2P
|
|
isCfg.ChainService = cfg.ChainService
|
|
|
|
rsCfg := DefaultRegularSyncConfig()
|
|
rsCfg.ChainService = cfg.ChainService
|
|
rsCfg.BeaconDB = cfg.BeaconDB
|
|
rsCfg.P2P = cfg.P2P
|
|
rsCfg.AttsService = cfg.AttsService
|
|
rsCfg.OperationService = cfg.OperationService
|
|
|
|
sq := NewQuerierService(ctx, sqCfg)
|
|
rs := NewRegularSyncService(ctx, rsCfg)
|
|
|
|
isCfg.SyncService = rs
|
|
is := initialsync.NewInitialSyncService(ctx, isCfg)
|
|
|
|
return &Service{
|
|
RegularSync: rs,
|
|
InitialSync: is,
|
|
Querier: sq,
|
|
}
|
|
|
|
}
|
|
|
|
// Start kicks off the sync service
|
|
func (ss *Service) Start() {
|
|
slog.Info("Starting service")
|
|
go ss.run()
|
|
}
|
|
|
|
// Stop ends all the currently running routines
|
|
// which are part of the sync service.
|
|
func (ss *Service) Stop() error {
|
|
err := ss.Querier.Stop()
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
err = ss.InitialSync.Stop()
|
|
if err != nil {
|
|
return err
|
|
}
|
|
return ss.RegularSync.Stop()
|
|
}
|
|
|
|
// Status checks the status of the node. It returns nil if it's synced
|
|
// with the rest of the network and no errors occurred. Otherwise, it returns an error.
|
|
func (ss *Service) Status() error {
|
|
synced, err := ss.Querier.IsSynced()
|
|
if !synced && err != nil {
|
|
return err
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (ss *Service) run() {
|
|
ss.Querier.Start()
|
|
synced, err := ss.Querier.IsSynced()
|
|
if err != nil {
|
|
slog.Fatalf("Unable to retrieve result from sync querier %v", err)
|
|
}
|
|
|
|
if synced {
|
|
ss.RegularSync.Start()
|
|
return
|
|
}
|
|
|
|
// Sets the highest observed slot from querier.
|
|
ss.InitialSync.InitializeObservedSlot(ss.Querier.currentHeadSlot)
|
|
|
|
// Sets the state root of the highest observed slot.
|
|
ss.InitialSync.InitializeStateRoot(ss.Querier.currentFinalizedStateRoot)
|
|
|
|
ss.InitialSync.Start()
|
|
}
|