mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
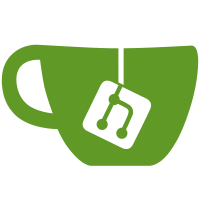
* Add overflow checks * More fixes * Typos * Merge branch 'master' into fix-overflow * One more regression test * Merge branch 'fix-overflow' of github.com:prysmaticlabs/prysm into fix-overflow * Gazelle * Merge branch 'master' into fix-overflow * Nishant's feedback * Merge refs/heads/master into fix-overflow
631 lines
21 KiB
Go
631 lines
21 KiB
Go
package helpers
|
|
|
|
import (
|
|
"fmt"
|
|
"reflect"
|
|
"strconv"
|
|
"testing"
|
|
|
|
ethpb "github.com/prysmaticlabs/ethereumapis/eth/v1alpha1"
|
|
"github.com/prysmaticlabs/go-bitfield"
|
|
beaconstate "github.com/prysmaticlabs/prysm/beacon-chain/state"
|
|
pb "github.com/prysmaticlabs/prysm/proto/beacon/p2p/v1"
|
|
"github.com/prysmaticlabs/prysm/shared/bytesutil"
|
|
"github.com/prysmaticlabs/prysm/shared/hashutil"
|
|
"github.com/prysmaticlabs/prysm/shared/params"
|
|
"github.com/prysmaticlabs/prysm/shared/sliceutil"
|
|
"github.com/prysmaticlabs/prysm/shared/testutil/assert"
|
|
"github.com/prysmaticlabs/prysm/shared/testutil/require"
|
|
)
|
|
|
|
func TestComputeCommittee_WithoutCache(t *testing.T) {
|
|
// Create 10 committees
|
|
committeeCount := uint64(10)
|
|
validatorCount := committeeCount * params.BeaconConfig().TargetCommitteeSize
|
|
validators := make([]*ethpb.Validator, validatorCount)
|
|
|
|
for i := 0; i < len(validators); i++ {
|
|
k := make([]byte, 48)
|
|
copy(k, strconv.Itoa(i))
|
|
validators[i] = ðpb.Validator{
|
|
PublicKey: k,
|
|
WithdrawalCredentials: make([]byte, 32),
|
|
ExitEpoch: params.BeaconConfig().FarFutureEpoch,
|
|
}
|
|
}
|
|
|
|
state, err := beaconstate.InitializeFromProto(&pb.BeaconState{
|
|
Validators: validators,
|
|
Slot: 200,
|
|
BlockRoots: make([][]byte, params.BeaconConfig().SlotsPerHistoricalRoot),
|
|
StateRoots: make([][]byte, params.BeaconConfig().SlotsPerHistoricalRoot),
|
|
RandaoMixes: make([][]byte, params.BeaconConfig().EpochsPerHistoricalVector),
|
|
})
|
|
require.NoError(t, err)
|
|
|
|
epoch := CurrentEpoch(state)
|
|
indices, err := ActiveValidatorIndices(state, epoch)
|
|
require.NoError(t, err)
|
|
seed, err := Seed(state, epoch, params.BeaconConfig().DomainBeaconAttester)
|
|
require.NoError(t, err)
|
|
committees, err := ComputeCommittee(indices, seed, 0, 1 /* Total committee*/)
|
|
assert.NoError(t, err, "Could not compute committee")
|
|
|
|
// Test shuffled indices are correct for index 5 committee
|
|
index := uint64(5)
|
|
committee5, err := ComputeCommittee(indices, seed, index, committeeCount)
|
|
assert.NoError(t, err, "Could not compute committee")
|
|
start := sliceutil.SplitOffset(validatorCount, committeeCount, index)
|
|
end := sliceutil.SplitOffset(validatorCount, committeeCount, index+1)
|
|
assert.DeepEqual(t, committee5, committees[start:end], "Committee has different shuffled indices")
|
|
|
|
// Test shuffled indices are correct for index 9 committee
|
|
index = uint64(9)
|
|
committee9, err := ComputeCommittee(indices, seed, index, committeeCount)
|
|
assert.NoError(t, err, "Could not compute committee")
|
|
start = sliceutil.SplitOffset(validatorCount, committeeCount, index)
|
|
end = sliceutil.SplitOffset(validatorCount, committeeCount, index+1)
|
|
assert.DeepEqual(t, committee9, committees[start:end], "Committee has different shuffled indices")
|
|
}
|
|
|
|
func TestComputeCommittee_RegressionTest(t *testing.T) {
|
|
indices := []uint64{1, 3, 8, 16, 18, 19, 20, 23, 30, 35, 43, 46, 47, 54, 56, 58, 69, 70, 71, 83, 84, 85, 91, 96, 100, 103, 105, 106, 112, 121, 127, 128, 129, 140, 142, 144, 146, 147, 149, 152, 153, 154, 157, 160, 173, 175, 180, 182, 188, 189, 191, 194, 201, 204, 217, 221, 226, 228, 230, 231, 239, 241, 249, 250, 255}
|
|
seed := [32]byte{68, 110, 161, 250, 98, 230, 161, 172, 227, 226, 99, 11, 138, 124, 201, 134, 38, 197, 0, 120, 6, 165, 122, 34, 19, 216, 43, 226, 210, 114, 165, 183}
|
|
index := uint64(215)
|
|
count := uint64(32)
|
|
_, err := ComputeCommittee(indices, seed, index, count)
|
|
require.ErrorContains(t, "index out of range", err)
|
|
}
|
|
|
|
func TestVerifyBitfieldLength_OK(t *testing.T) {
|
|
bf := bitfield.Bitlist{0xFF, 0x01}
|
|
committeeSize := uint64(8)
|
|
assert.NoError(t, VerifyBitfieldLength(bf, committeeSize), "Bitfield is not validated when it was supposed to be")
|
|
|
|
bf = bitfield.Bitlist{0xFF, 0x07}
|
|
committeeSize = 10
|
|
assert.NoError(t, VerifyBitfieldLength(bf, committeeSize), "Bitfield is not validated when it was supposed to be")
|
|
}
|
|
|
|
func TestCommitteeAssignments_CannotRetrieveFutureEpoch(t *testing.T) {
|
|
ClearCache()
|
|
epoch := uint64(1)
|
|
state, err := beaconstate.InitializeFromProto(&pb.BeaconState{
|
|
Slot: 0, // Epoch 0.
|
|
})
|
|
require.NoError(t, err)
|
|
_, _, err = CommitteeAssignments(state, epoch+1)
|
|
assert.ErrorContains(t, "can't be greater than next epoch", err)
|
|
}
|
|
|
|
func TestCommitteeAssignments_NoProposerForSlot0(t *testing.T) {
|
|
validators := make([]*ethpb.Validator, 4*params.BeaconConfig().SlotsPerEpoch)
|
|
for i := 0; i < len(validators); i++ {
|
|
var activationEpoch uint64
|
|
if i >= len(validators)/2 {
|
|
activationEpoch = 3
|
|
}
|
|
validators[i] = ðpb.Validator{
|
|
ActivationEpoch: activationEpoch,
|
|
ExitEpoch: params.BeaconConfig().FarFutureEpoch,
|
|
}
|
|
}
|
|
state, err := beaconstate.InitializeFromProto(&pb.BeaconState{
|
|
Validators: validators,
|
|
Slot: 2 * params.BeaconConfig().SlotsPerEpoch, // epoch 2
|
|
RandaoMixes: make([][]byte, params.BeaconConfig().EpochsPerHistoricalVector),
|
|
})
|
|
require.NoError(t, err)
|
|
ClearCache()
|
|
_, proposerIndexToSlots, err := CommitteeAssignments(state, 0)
|
|
require.NoError(t, err, "Failed to determine CommitteeAssignments")
|
|
for _, slots := range proposerIndexToSlots {
|
|
for _, s := range slots {
|
|
assert.NotEqual(t, uint64(0), s, "No proposer should be assigned to slot 0")
|
|
}
|
|
}
|
|
}
|
|
|
|
func TestCommitteeAssignments_CanRetrieve(t *testing.T) {
|
|
// Initialize test with 256 validators, each slot and each index gets 4 validators.
|
|
validators := make([]*ethpb.Validator, 4*params.BeaconConfig().SlotsPerEpoch)
|
|
for i := 0; i < len(validators); i++ {
|
|
// First 2 epochs only half validators are activated.
|
|
var activationEpoch uint64
|
|
if i >= len(validators)/2 {
|
|
activationEpoch = 3
|
|
}
|
|
validators[i] = ðpb.Validator{
|
|
ActivationEpoch: activationEpoch,
|
|
ExitEpoch: params.BeaconConfig().FarFutureEpoch,
|
|
}
|
|
}
|
|
|
|
state, err := beaconstate.InitializeFromProto(&pb.BeaconState{
|
|
Validators: validators,
|
|
Slot: 2 * params.BeaconConfig().SlotsPerEpoch, // epoch 2
|
|
RandaoMixes: make([][]byte, params.BeaconConfig().EpochsPerHistoricalVector),
|
|
})
|
|
require.NoError(t, err)
|
|
|
|
tests := []struct {
|
|
index uint64
|
|
slot uint64
|
|
committee []uint64
|
|
committeeIndex uint64
|
|
isProposer bool
|
|
proposerSlot uint64
|
|
}{
|
|
{
|
|
index: 0,
|
|
slot: 78,
|
|
committee: []uint64{0, 38},
|
|
committeeIndex: 0,
|
|
isProposer: false,
|
|
},
|
|
{
|
|
index: 1,
|
|
slot: 71,
|
|
committee: []uint64{1, 4},
|
|
committeeIndex: 0,
|
|
isProposer: true,
|
|
proposerSlot: 79,
|
|
},
|
|
{
|
|
index: 11,
|
|
slot: 90,
|
|
committee: []uint64{31, 11},
|
|
committeeIndex: 0,
|
|
isProposer: false,
|
|
}, {
|
|
index: 2,
|
|
slot: 127, // 3rd epoch has more active validators
|
|
committee: []uint64{89, 2, 81, 5},
|
|
committeeIndex: 0,
|
|
isProposer: false,
|
|
},
|
|
}
|
|
|
|
for i, tt := range tests {
|
|
t.Run(fmt.Sprintf("%d", i), func(t *testing.T) {
|
|
ClearCache()
|
|
validatorIndexToCommittee, proposerIndexToSlots, err := CommitteeAssignments(state, SlotToEpoch(tt.slot))
|
|
require.NoError(t, err, "Failed to determine CommitteeAssignments")
|
|
cac := validatorIndexToCommittee[tt.index]
|
|
assert.Equal(t, tt.committeeIndex, cac.CommitteeIndex, "Unexpected committeeIndex for validator index %d", tt.index)
|
|
assert.Equal(t, tt.slot, cac.AttesterSlot, "Unexpected slot for validator index %d", tt.index)
|
|
if len(proposerIndexToSlots[tt.index]) > 0 && proposerIndexToSlots[tt.index][0] != tt.proposerSlot {
|
|
t.Errorf("wanted proposer slot %d, got proposer slot %d for validator index %d",
|
|
tt.proposerSlot, proposerIndexToSlots[tt.index][0], tt.index)
|
|
}
|
|
assert.DeepEqual(t, tt.committee, cac.Committee, "Unexpected committee for validator index %d", tt.index)
|
|
})
|
|
}
|
|
}
|
|
|
|
func TestCommitteeAssignments_EverySlotHasMin1Proposer(t *testing.T) {
|
|
// Initialize test with 256 validators, each slot and each index gets 4 validators.
|
|
validators := make([]*ethpb.Validator, 4*params.BeaconConfig().SlotsPerEpoch)
|
|
for i := 0; i < len(validators); i++ {
|
|
validators[i] = ðpb.Validator{
|
|
ActivationEpoch: 0,
|
|
ExitEpoch: params.BeaconConfig().FarFutureEpoch,
|
|
}
|
|
}
|
|
state, err := beaconstate.InitializeFromProto(&pb.BeaconState{
|
|
Validators: validators,
|
|
Slot: 2 * params.BeaconConfig().SlotsPerEpoch, // epoch 2
|
|
RandaoMixes: make([][]byte, params.BeaconConfig().EpochsPerHistoricalVector),
|
|
})
|
|
require.NoError(t, err)
|
|
ClearCache()
|
|
epoch := uint64(1)
|
|
_, proposerIndexToSlots, err := CommitteeAssignments(state, epoch)
|
|
require.NoError(t, err, "Failed to determine CommitteeAssignments")
|
|
|
|
slotsWithProposers := make(map[uint64]bool)
|
|
for _, proposerSlots := range proposerIndexToSlots {
|
|
for _, slot := range proposerSlots {
|
|
slotsWithProposers[slot] = true
|
|
}
|
|
}
|
|
assert.Equal(t, params.BeaconConfig().SlotsPerEpoch, uint64(len(slotsWithProposers)), "Unexpected slots")
|
|
startSlot, err := StartSlot(epoch)
|
|
require.NoError(t, err)
|
|
endSlot, err := StartSlot(epoch + 1)
|
|
require.NoError(t, err)
|
|
for i := startSlot; i < endSlot; i++ {
|
|
hasProposer := slotsWithProposers[i]
|
|
assert.Equal(t, true, hasProposer, "Expected every slot in epoch 1 to have a proposer, slot %d did not", i)
|
|
}
|
|
}
|
|
|
|
func TestVerifyAttestationBitfieldLengths_OK(t *testing.T) {
|
|
validators := make([]*ethpb.Validator, 2*params.BeaconConfig().SlotsPerEpoch)
|
|
activeRoots := make([][]byte, params.BeaconConfig().EpochsPerHistoricalVector)
|
|
for i := 0; i < len(validators); i++ {
|
|
validators[i] = ðpb.Validator{
|
|
ExitEpoch: params.BeaconConfig().FarFutureEpoch,
|
|
}
|
|
}
|
|
|
|
state, err := beaconstate.InitializeFromProto(&pb.BeaconState{
|
|
Validators: validators,
|
|
RandaoMixes: activeRoots,
|
|
})
|
|
require.NoError(t, err)
|
|
|
|
tests := []struct {
|
|
attestation *ethpb.Attestation
|
|
stateSlot uint64
|
|
verificationFailure bool
|
|
}{
|
|
{
|
|
attestation: ðpb.Attestation{
|
|
AggregationBits: bitfield.Bitlist{0x05},
|
|
Data: ðpb.AttestationData{
|
|
CommitteeIndex: 5,
|
|
Target: ðpb.Checkpoint{Root: make([]byte, 32)},
|
|
},
|
|
},
|
|
stateSlot: 5,
|
|
},
|
|
{
|
|
|
|
attestation: ðpb.Attestation{
|
|
AggregationBits: bitfield.Bitlist{0x06},
|
|
Data: ðpb.AttestationData{
|
|
CommitteeIndex: 10,
|
|
Target: ðpb.Checkpoint{Root: make([]byte, 32)},
|
|
},
|
|
},
|
|
stateSlot: 10,
|
|
},
|
|
{
|
|
attestation: ðpb.Attestation{
|
|
AggregationBits: bitfield.Bitlist{0x06},
|
|
Data: ðpb.AttestationData{
|
|
CommitteeIndex: 20,
|
|
Target: ðpb.Checkpoint{Root: make([]byte, 32)},
|
|
},
|
|
},
|
|
stateSlot: 20,
|
|
},
|
|
{
|
|
attestation: ðpb.Attestation{
|
|
AggregationBits: bitfield.Bitlist{0x06},
|
|
Data: ðpb.AttestationData{
|
|
CommitteeIndex: 20,
|
|
Target: ðpb.Checkpoint{Root: make([]byte, 32)},
|
|
},
|
|
},
|
|
stateSlot: 20,
|
|
},
|
|
{
|
|
attestation: ðpb.Attestation{
|
|
AggregationBits: bitfield.Bitlist{0xFF, 0xC0, 0x01},
|
|
Data: ðpb.AttestationData{
|
|
CommitteeIndex: 5,
|
|
Target: ðpb.Checkpoint{Root: make([]byte, 32)},
|
|
},
|
|
},
|
|
stateSlot: 5,
|
|
verificationFailure: true,
|
|
},
|
|
{
|
|
attestation: ðpb.Attestation{
|
|
AggregationBits: bitfield.Bitlist{0xFF, 0x01},
|
|
Data: ðpb.AttestationData{
|
|
CommitteeIndex: 20,
|
|
Target: ðpb.Checkpoint{Root: make([]byte, 32)},
|
|
},
|
|
},
|
|
stateSlot: 20,
|
|
verificationFailure: true,
|
|
},
|
|
}
|
|
|
|
for i, tt := range tests {
|
|
ClearCache()
|
|
require.NoError(t, state.SetSlot(tt.stateSlot))
|
|
err := VerifyAttestationBitfieldLengths(state, tt.attestation)
|
|
if tt.verificationFailure {
|
|
assert.NotNil(t, err, "Verification succeeded when it was supposed to fail")
|
|
} else {
|
|
assert.NoError(t, err, "%d Failed to verify bitfield: %v", i, err)
|
|
}
|
|
}
|
|
}
|
|
|
|
func TestShuffledIndices_ShuffleRightLength(t *testing.T) {
|
|
valiatorCount := 1000
|
|
validators := make([]*ethpb.Validator, valiatorCount)
|
|
indices := make([]uint64, valiatorCount)
|
|
for i := 0; i < valiatorCount; i++ {
|
|
validators[i] = ðpb.Validator{
|
|
ExitEpoch: params.BeaconConfig().FarFutureEpoch,
|
|
}
|
|
indices[i] = uint64(i)
|
|
}
|
|
state, err := beaconstate.InitializeFromProto(&pb.BeaconState{
|
|
Validators: validators,
|
|
RandaoMixes: make([][]byte, params.BeaconConfig().EpochsPerHistoricalVector),
|
|
})
|
|
require.NoError(t, err)
|
|
// Test for current epoch
|
|
shuffledIndices, err := ShuffledIndices(state, 0)
|
|
require.NoError(t, err)
|
|
assert.Equal(t, valiatorCount, len(shuffledIndices), "Incorrect shuffled indices count")
|
|
if reflect.DeepEqual(indices, shuffledIndices) {
|
|
t.Error("Shuffling did not happen")
|
|
}
|
|
|
|
// Test for next epoch
|
|
shuffledIndices, err = ShuffledIndices(state, 1)
|
|
require.NoError(t, err)
|
|
assert.Equal(t, valiatorCount, len(shuffledIndices), "Incorrect shuffled indices count")
|
|
if reflect.DeepEqual(indices, shuffledIndices) {
|
|
t.Error("Shuffling did not happen")
|
|
}
|
|
}
|
|
|
|
func TestUpdateCommitteeCache_CanUpdate(t *testing.T) {
|
|
ClearCache()
|
|
validatorCount := params.BeaconConfig().MinGenesisActiveValidatorCount
|
|
validators := make([]*ethpb.Validator, validatorCount)
|
|
indices := make([]uint64, validatorCount)
|
|
for i := uint64(0); i < validatorCount; i++ {
|
|
validators[i] = ðpb.Validator{
|
|
ExitEpoch: params.BeaconConfig().FarFutureEpoch,
|
|
}
|
|
indices[i] = i
|
|
}
|
|
state, err := beaconstate.InitializeFromProto(&pb.BeaconState{
|
|
Validators: validators,
|
|
RandaoMixes: make([][]byte, params.BeaconConfig().EpochsPerHistoricalVector),
|
|
})
|
|
require.NoError(t, err)
|
|
require.NoError(t, UpdateCommitteeCache(state, CurrentEpoch(state)))
|
|
|
|
epoch := uint64(1)
|
|
idx := uint64(1)
|
|
seed, err := Seed(state, epoch, params.BeaconConfig().DomainBeaconAttester)
|
|
require.NoError(t, err)
|
|
|
|
indices, err = committeeCache.Committee(epoch*params.BeaconConfig().SlotsPerEpoch, seed, idx)
|
|
require.NoError(t, err)
|
|
assert.Equal(t, params.BeaconConfig().TargetCommitteeSize, uint64(len(indices)), "Did not save correct indices lengths")
|
|
}
|
|
|
|
func BenchmarkComputeCommittee300000_WithPreCache(b *testing.B) {
|
|
validators := make([]*ethpb.Validator, 300000)
|
|
for i := 0; i < len(validators); i++ {
|
|
validators[i] = ðpb.Validator{
|
|
ExitEpoch: params.BeaconConfig().FarFutureEpoch,
|
|
}
|
|
}
|
|
state, err := beaconstate.InitializeFromProto(&pb.BeaconState{
|
|
Validators: validators,
|
|
RandaoMixes: make([][]byte, params.BeaconConfig().EpochsPerHistoricalVector),
|
|
})
|
|
require.NoError(b, err)
|
|
|
|
epoch := CurrentEpoch(state)
|
|
indices, err := ActiveValidatorIndices(state, epoch)
|
|
require.NoError(b, err)
|
|
seed, err := Seed(state, epoch, params.BeaconConfig().DomainBeaconAttester)
|
|
require.NoError(b, err)
|
|
|
|
index := uint64(3)
|
|
_, err = ComputeCommittee(indices, seed, index, params.BeaconConfig().MaxCommitteesPerSlot)
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
|
|
b.ResetTimer()
|
|
for n := 0; n < b.N; n++ {
|
|
_, err := ComputeCommittee(indices, seed, index, params.BeaconConfig().MaxCommitteesPerSlot)
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
}
|
|
}
|
|
|
|
func BenchmarkComputeCommittee3000000_WithPreCache(b *testing.B) {
|
|
validators := make([]*ethpb.Validator, 3000000)
|
|
for i := 0; i < len(validators); i++ {
|
|
validators[i] = ðpb.Validator{
|
|
ExitEpoch: params.BeaconConfig().FarFutureEpoch,
|
|
}
|
|
}
|
|
state, err := beaconstate.InitializeFromProto(&pb.BeaconState{
|
|
Validators: validators,
|
|
RandaoMixes: make([][]byte, params.BeaconConfig().EpochsPerHistoricalVector),
|
|
})
|
|
require.NoError(b, err)
|
|
|
|
epoch := CurrentEpoch(state)
|
|
indices, err := ActiveValidatorIndices(state, epoch)
|
|
require.NoError(b, err)
|
|
seed, err := Seed(state, epoch, params.BeaconConfig().DomainBeaconAttester)
|
|
require.NoError(b, err)
|
|
|
|
index := uint64(3)
|
|
_, err = ComputeCommittee(indices, seed, index, params.BeaconConfig().MaxCommitteesPerSlot)
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
|
|
b.ResetTimer()
|
|
for n := 0; n < b.N; n++ {
|
|
_, err := ComputeCommittee(indices, seed, index, params.BeaconConfig().MaxCommitteesPerSlot)
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
}
|
|
}
|
|
|
|
func BenchmarkComputeCommittee128000_WithOutPreCache(b *testing.B) {
|
|
validators := make([]*ethpb.Validator, 128000)
|
|
for i := 0; i < len(validators); i++ {
|
|
validators[i] = ðpb.Validator{
|
|
ExitEpoch: params.BeaconConfig().FarFutureEpoch,
|
|
}
|
|
}
|
|
state, err := beaconstate.InitializeFromProto(&pb.BeaconState{
|
|
Validators: validators,
|
|
RandaoMixes: make([][]byte, params.BeaconConfig().EpochsPerHistoricalVector),
|
|
})
|
|
require.NoError(b, err)
|
|
|
|
epoch := CurrentEpoch(state)
|
|
indices, err := ActiveValidatorIndices(state, epoch)
|
|
require.NoError(b, err)
|
|
seed, err := Seed(state, epoch, params.BeaconConfig().DomainBeaconAttester)
|
|
require.NoError(b, err)
|
|
|
|
i := uint64(0)
|
|
index := uint64(0)
|
|
b.ResetTimer()
|
|
for n := 0; n < b.N; n++ {
|
|
i++
|
|
_, err := ComputeCommittee(indices, seed, index, params.BeaconConfig().MaxCommitteesPerSlot)
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
if i < params.BeaconConfig().TargetCommitteeSize {
|
|
index = (index + 1) % params.BeaconConfig().MaxCommitteesPerSlot
|
|
i = 0
|
|
}
|
|
}
|
|
}
|
|
|
|
func BenchmarkComputeCommittee1000000_WithOutCache(b *testing.B) {
|
|
validators := make([]*ethpb.Validator, 1000000)
|
|
for i := 0; i < len(validators); i++ {
|
|
validators[i] = ðpb.Validator{
|
|
ExitEpoch: params.BeaconConfig().FarFutureEpoch,
|
|
}
|
|
}
|
|
state, err := beaconstate.InitializeFromProto(&pb.BeaconState{
|
|
Validators: validators,
|
|
RandaoMixes: make([][]byte, params.BeaconConfig().EpochsPerHistoricalVector),
|
|
})
|
|
require.NoError(b, err)
|
|
|
|
epoch := CurrentEpoch(state)
|
|
indices, err := ActiveValidatorIndices(state, epoch)
|
|
require.NoError(b, err)
|
|
seed, err := Seed(state, epoch, params.BeaconConfig().DomainBeaconAttester)
|
|
require.NoError(b, err)
|
|
|
|
i := uint64(0)
|
|
index := uint64(0)
|
|
b.ResetTimer()
|
|
for n := 0; n < b.N; n++ {
|
|
i++
|
|
_, err := ComputeCommittee(indices, seed, index, params.BeaconConfig().MaxCommitteesPerSlot)
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
if i < params.BeaconConfig().TargetCommitteeSize {
|
|
index = (index + 1) % params.BeaconConfig().MaxCommitteesPerSlot
|
|
i = 0
|
|
}
|
|
}
|
|
}
|
|
|
|
func BenchmarkComputeCommittee4000000_WithOutCache(b *testing.B) {
|
|
validators := make([]*ethpb.Validator, 4000000)
|
|
for i := 0; i < len(validators); i++ {
|
|
validators[i] = ðpb.Validator{
|
|
ExitEpoch: params.BeaconConfig().FarFutureEpoch,
|
|
}
|
|
}
|
|
state, err := beaconstate.InitializeFromProto(&pb.BeaconState{
|
|
Validators: validators,
|
|
RandaoMixes: make([][]byte, params.BeaconConfig().EpochsPerHistoricalVector),
|
|
})
|
|
require.NoError(b, err)
|
|
|
|
epoch := CurrentEpoch(state)
|
|
indices, err := ActiveValidatorIndices(state, epoch)
|
|
require.NoError(b, err)
|
|
seed, err := Seed(state, epoch, params.BeaconConfig().DomainBeaconAttester)
|
|
require.NoError(b, err)
|
|
|
|
i := uint64(0)
|
|
index := uint64(0)
|
|
b.ResetTimer()
|
|
for n := 0; n < b.N; n++ {
|
|
i++
|
|
_, err := ComputeCommittee(indices, seed, index, params.BeaconConfig().MaxCommitteesPerSlot)
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
if i < params.BeaconConfig().TargetCommitteeSize {
|
|
index = (index + 1) % params.BeaconConfig().MaxCommitteesPerSlot
|
|
i = 0
|
|
}
|
|
}
|
|
}
|
|
|
|
func TestBeaconCommitteeFromState_UpdateCacheForPreviousEpoch(t *testing.T) {
|
|
committeeSize := uint64(16)
|
|
validators := make([]*ethpb.Validator, committeeSize*params.BeaconConfig().SlotsPerEpoch)
|
|
for i := 0; i < len(validators); i++ {
|
|
validators[i] = ðpb.Validator{
|
|
ExitEpoch: params.BeaconConfig().FarFutureEpoch,
|
|
}
|
|
}
|
|
|
|
state, err := beaconstate.InitializeFromProto(&pb.BeaconState{
|
|
Slot: params.BeaconConfig().SlotsPerEpoch,
|
|
Validators: validators,
|
|
RandaoMixes: make([][]byte, params.BeaconConfig().EpochsPerHistoricalVector),
|
|
})
|
|
require.NoError(t, err)
|
|
_, err = BeaconCommitteeFromState(state, 1 /* previous epoch */, 0)
|
|
require.NoError(t, err)
|
|
|
|
// Verify previous epoch is cached
|
|
seed, err := Seed(state, 0, params.BeaconConfig().DomainBeaconAttester)
|
|
require.NoError(t, err)
|
|
activeIndices, err := committeeCache.ActiveIndices(seed)
|
|
require.NoError(t, err)
|
|
assert.NotNil(t, activeIndices, "Did not cache active indices")
|
|
}
|
|
|
|
func TestPrecomputeProposerIndices_Ok(t *testing.T) {
|
|
validators := make([]*ethpb.Validator, params.BeaconConfig().MinGenesisActiveValidatorCount)
|
|
for i := 0; i < len(validators); i++ {
|
|
validators[i] = ðpb.Validator{
|
|
ExitEpoch: params.BeaconConfig().FarFutureEpoch,
|
|
}
|
|
}
|
|
|
|
state, err := beaconstate.InitializeFromProto(&pb.BeaconState{
|
|
Validators: validators,
|
|
RandaoMixes: make([][]byte, params.BeaconConfig().EpochsPerHistoricalVector),
|
|
})
|
|
require.NoError(t, err)
|
|
|
|
indices, err := ActiveValidatorIndices(state, 0)
|
|
require.NoError(t, err)
|
|
|
|
proposerIndices, err := precomputeProposerIndices(state, indices)
|
|
require.NoError(t, err)
|
|
|
|
var wantedProposerIndices []uint64
|
|
seed, err := Seed(state, 0, params.BeaconConfig().DomainBeaconProposer)
|
|
require.NoError(t, err)
|
|
for i := uint64(0); i < params.BeaconConfig().SlotsPerEpoch; i++ {
|
|
seedWithSlot := append(seed[:], bytesutil.Bytes8(i)...)
|
|
seedWithSlotHash := hashutil.Hash(seedWithSlot)
|
|
index, err := ComputeProposerIndex(state, indices, seedWithSlotHash)
|
|
require.NoError(t, err)
|
|
wantedProposerIndices = append(wantedProposerIndices, index)
|
|
}
|
|
assert.DeepEqual(t, wantedProposerIndices, proposerIndices, "Did not precompute proposer indices correctly")
|
|
}
|