mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 12:57:18 +00:00
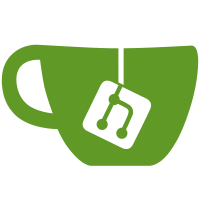
* Add REST implementation for Validator's WaitForChainStart * Add missing error mapping * Add missing bazel dependency * Add missing tests * Address PR comments * Replace EventErrorJson with DefaultErrorJson * Add tests for WaitForChainStart * Refactor tests * Address PR comments * Add gazelle:build_tag use_beacon_api comment in BUILD.bazel * Address PR comments * Address PR comments Co-authored-by: Radosław Kapka <rkapka@wp.pl>
90 lines
2.0 KiB
Go
90 lines
2.0 KiB
Go
//go:build use_beacon_api
|
|
// +build use_beacon_api
|
|
|
|
package beacon_api
|
|
|
|
import (
|
|
"encoding/json"
|
|
"net/http"
|
|
|
|
"github.com/prysmaticlabs/prysm/v3/api/gateway/apimiddleware"
|
|
rpcmiddleware "github.com/prysmaticlabs/prysm/v3/beacon-chain/rpc/apimiddleware"
|
|
)
|
|
|
|
func internalServerErrHandler(w http.ResponseWriter, r *http.Request) {
|
|
internalErrorJson := &apimiddleware.DefaultErrorJson{
|
|
Code: http.StatusInternalServerError,
|
|
Message: "Internal server error",
|
|
}
|
|
|
|
marshalledError, err := json.Marshal(internalErrorJson)
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
|
|
w.WriteHeader(http.StatusInternalServerError)
|
|
_, err = w.Write(marshalledError)
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
}
|
|
|
|
func notFoundErrHandler(w http.ResponseWriter, r *http.Request) {
|
|
internalErrorJson := &apimiddleware.DefaultErrorJson{
|
|
Code: http.StatusNotFound,
|
|
Message: "Not found",
|
|
}
|
|
|
|
marshalledError, err := json.Marshal(internalErrorJson)
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
|
|
w.WriteHeader(http.StatusNotFound)
|
|
_, err = w.Write(marshalledError)
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
}
|
|
|
|
func invalidErr999Handler(w http.ResponseWriter, r *http.Request) {
|
|
internalErrorJson := &apimiddleware.DefaultErrorJson{
|
|
Code: 999,
|
|
Message: "Invalid error",
|
|
}
|
|
|
|
marshalledError, err := json.Marshal(internalErrorJson)
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
|
|
w.WriteHeader(999)
|
|
_, err = w.Write(marshalledError)
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
}
|
|
|
|
func invalidJsonErrHandler(w http.ResponseWriter, r *http.Request) {
|
|
w.WriteHeader(http.StatusNotFound)
|
|
_, err := w.Write([]byte("foo"))
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
}
|
|
|
|
func createGenesisHandler(data *rpcmiddleware.GenesisResponse_GenesisJson) http.HandlerFunc {
|
|
return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) {
|
|
genesisResponseJson := &rpcmiddleware.GenesisResponseJson{Data: data}
|
|
marshalledResponse, err := json.Marshal(genesisResponseJson)
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
|
|
_, err = w.Write(marshalledResponse)
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
})
|
|
}
|