mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2025-01-08 10:41:19 +00:00
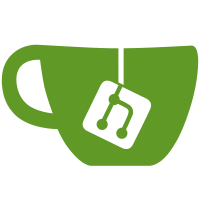
Former-commit-id: 44c0c5682c0296d94943b354171e69b4c8cf5312 [formerly 54da5cb45b098f0737fb3c37964e612dfe93c751] Former-commit-id: 5d84d3c5038a01c4108e519d5a5c69033ace8ae2
46 lines
1.3 KiB
Go
46 lines
1.3 KiB
Go
// Package notary defines all relevant functionality for a Notary actor
|
|
// within a sharded Ethereum blockchain.
|
|
package notary
|
|
|
|
import (
|
|
"github.com/ethereum/go-ethereum/ethdb"
|
|
"github.com/ethereum/go-ethereum/log"
|
|
"github.com/ethereum/go-ethereum/sharding"
|
|
"github.com/ethereum/go-ethereum/sharding/mainchain"
|
|
)
|
|
|
|
// Notary holds functionality required to run a collation notary
|
|
// in a sharded system. Must satisfy the Service interface defined in
|
|
// sharding/service.go.
|
|
type Notary struct {
|
|
smcClient *mainchain.SMCClient
|
|
shardp2p sharding.ShardP2P
|
|
shardChainDb ethdb.Database
|
|
}
|
|
|
|
// NewNotary creates a new notary instance.
|
|
func NewNotary(smcClient *mainchain.SMCClient, shardp2p sharding.ShardP2P, shardChainDb ethdb.Database) (*Notary, error) {
|
|
return &Notary{smcClient, shardp2p, shardChainDb}, nil
|
|
}
|
|
|
|
// Start the main routine for a notary.
|
|
func (n *Notary) Start() error {
|
|
log.Info("Starting notary service")
|
|
|
|
// TODO: handle this better through goroutines. Right now, these methods
|
|
// are blocking.
|
|
if n.smcClient.DepositFlag() {
|
|
if err := joinNotaryPool(n.smcClient); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
|
|
return subscribeBlockHeaders(n.smcClient)
|
|
}
|
|
|
|
// Stop the main loop for notarizing collations.
|
|
func (n *Notary) Stop() error {
|
|
log.Info("Stopping notary service")
|
|
return nil
|
|
}
|