mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-29 06:37:17 +00:00
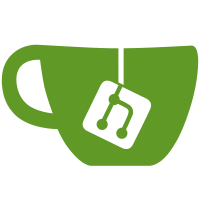
* Update kv aggregated_test.go * Update block_test.go * Update forkchoice_test.go * Update unaggregated_test.go * Update prepare_forkchoice_test.go * Update prune_expired_test.go * Update atts service_test.go * Update service_attester_test.go * Update service_proposer_test.go * Upate exit service_test.go * Gaz * Merge branch 'master' of github.com:prysmaticlabs/prysm * Move averageBalance from log.go to info.go * Move avg balance from log.go to info.go * Add info test * Remove unused logEpochData in log.go * Gaz * Merge branch 'master' of github.com:prysmaticlabs/prysm * Merge branch 'master' of github.com:prysmaticlabs/prysm * aggregation tests * attestation util tests * bench util tests * block util tests * herumi tests * bytesutil tests * Merge refs/heads/master into testutil-shared * Merge refs/heads/master into testutil-shared * Merge refs/heads/master into testutil-shared * Merge refs/heads/master into testutil-shared * Merge refs/heads/master into testutil-shared * Update shared/aggregation/attestations/attestations_test.go Co-authored-by: Victor Farazdagi <simple.square@gmail.com> * Merge branch 'master' of github.com:prysmaticlabs/prysm into testutil-shared * Fixed ordering * Merge branch 'testutil-shared' of github.com:prysmaticlabs/prysm into testutil-shared * Update shared/aggregation/attestations/attestations_test.go Co-authored-by: Victor Farazdagi <simple.square@gmail.com> * Update shared/bytesutil/bytes_test.go Co-authored-by: Victor Farazdagi <simple.square@gmail.com>
91 lines
3.1 KiB
Go
91 lines
3.1 KiB
Go
package blockutil
|
|
|
|
import (
|
|
"testing"
|
|
|
|
eth "github.com/prysmaticlabs/ethereumapis/eth/v1alpha1"
|
|
"github.com/prysmaticlabs/prysm/beacon-chain/state/stateutil"
|
|
"github.com/prysmaticlabs/prysm/shared/bytesutil"
|
|
"github.com/prysmaticlabs/prysm/shared/params"
|
|
"github.com/prysmaticlabs/prysm/shared/testutil/assert"
|
|
"github.com/prysmaticlabs/prysm/shared/testutil/require"
|
|
)
|
|
|
|
func TestBeaconBlockHeaderFromBlock(t *testing.T) {
|
|
hashLen := 32
|
|
blk := ð.BeaconBlock{
|
|
Slot: 200,
|
|
ProposerIndex: 2,
|
|
ParentRoot: bytesutil.PadTo([]byte("parent root"), hashLen),
|
|
StateRoot: bytesutil.PadTo([]byte("state root"), hashLen),
|
|
Body: ð.BeaconBlockBody{
|
|
Eth1Data: ð.Eth1Data{
|
|
BlockHash: bytesutil.PadTo([]byte("block hash"), hashLen),
|
|
DepositRoot: bytesutil.PadTo([]byte("deposit root"), hashLen),
|
|
DepositCount: 1,
|
|
},
|
|
RandaoReveal: bytesutil.PadTo([]byte("randao"), params.BeaconConfig().BLSSignatureLength),
|
|
Graffiti: bytesutil.PadTo([]byte("teehee"), hashLen),
|
|
ProposerSlashings: []*eth.ProposerSlashing{},
|
|
AttesterSlashings: []*eth.AttesterSlashing{},
|
|
Attestations: []*eth.Attestation{},
|
|
Deposits: []*eth.Deposit{},
|
|
VoluntaryExits: []*eth.SignedVoluntaryExit{},
|
|
},
|
|
}
|
|
bodyRoot, err := stateutil.BlockBodyRoot(blk.Body)
|
|
require.NoError(t, err)
|
|
want := ð.BeaconBlockHeader{
|
|
Slot: blk.Slot,
|
|
ProposerIndex: blk.ProposerIndex,
|
|
ParentRoot: blk.ParentRoot,
|
|
StateRoot: blk.StateRoot,
|
|
BodyRoot: bodyRoot[:],
|
|
}
|
|
|
|
bh, err := BeaconBlockHeaderFromBlock(blk)
|
|
require.NoError(t, err)
|
|
assert.DeepEqual(t, want, bh)
|
|
}
|
|
|
|
func TestSignedBeaconBlockHeaderFromBlock(t *testing.T) {
|
|
hashLen := 32
|
|
blk := ð.SignedBeaconBlock{Block: ð.BeaconBlock{
|
|
Slot: 200,
|
|
ProposerIndex: 2,
|
|
ParentRoot: bytesutil.PadTo([]byte("parent root"), hashLen),
|
|
StateRoot: bytesutil.PadTo([]byte("state root"), hashLen),
|
|
Body: ð.BeaconBlockBody{
|
|
Eth1Data: ð.Eth1Data{
|
|
BlockHash: bytesutil.PadTo([]byte("block hash"), hashLen),
|
|
DepositRoot: bytesutil.PadTo([]byte("deposit root"), hashLen),
|
|
DepositCount: 1,
|
|
},
|
|
RandaoReveal: bytesutil.PadTo([]byte("randao"), params.BeaconConfig().BLSSignatureLength),
|
|
Graffiti: bytesutil.PadTo([]byte("teehee"), hashLen),
|
|
ProposerSlashings: []*eth.ProposerSlashing{},
|
|
AttesterSlashings: []*eth.AttesterSlashing{},
|
|
Attestations: []*eth.Attestation{},
|
|
Deposits: []*eth.Deposit{},
|
|
VoluntaryExits: []*eth.SignedVoluntaryExit{},
|
|
},
|
|
},
|
|
Signature: bytesutil.PadTo([]byte("signature"), params.BeaconConfig().BLSSignatureLength),
|
|
}
|
|
bodyRoot, err := stateutil.BlockBodyRoot(blk.Block.Body)
|
|
require.NoError(t, err)
|
|
want := ð.SignedBeaconBlockHeader{Header: ð.BeaconBlockHeader{
|
|
Slot: blk.Block.Slot,
|
|
ProposerIndex: blk.Block.ProposerIndex,
|
|
ParentRoot: blk.Block.ParentRoot,
|
|
StateRoot: blk.Block.StateRoot,
|
|
BodyRoot: bodyRoot[:],
|
|
},
|
|
Signature: blk.Signature,
|
|
}
|
|
|
|
bh, err := SignedBeaconBlockHeaderFromBlock(blk)
|
|
require.NoError(t, err)
|
|
assert.DeepEqual(t, want, bh)
|
|
}
|