mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2025-01-09 11:11:20 +00:00
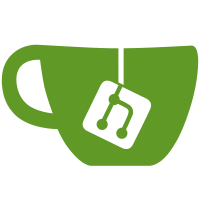
sharding: simplify service registry to prevent nil pointer errors Former-commit-id: ba4833c385e5212723932491810baad62e3ff0f9 [formerly c550c6d0837999f46a6de55a36fb1ae92d2ecd6f] Former-commit-id: 80e9e13bc811444b461dad6bdf9eec633b911bec
46 lines
1.4 KiB
Go
46 lines
1.4 KiB
Go
// Package observer launches a service attached to the sharding node
|
|
// that simply observes activity across the sharded Ethereum network.
|
|
package observer
|
|
|
|
import (
|
|
"context"
|
|
"fmt"
|
|
|
|
"github.com/ethereum/go-ethereum/log"
|
|
"github.com/ethereum/go-ethereum/sharding/database"
|
|
"github.com/ethereum/go-ethereum/sharding/p2p"
|
|
)
|
|
|
|
// Observer holds functionality required to run an observer service
|
|
// in a sharded system. Must satisfy the Service interface defined in
|
|
// sharding/service.go.
|
|
type Observer struct {
|
|
p2p *p2p.Server
|
|
dbService *database.ShardDB
|
|
shardID int
|
|
ctx context.Context
|
|
cancel context.CancelFunc
|
|
}
|
|
|
|
// NewObserver creates a struct instance of a observer service,
|
|
// it will have access to a p2p server and a shardChainDB.
|
|
func NewObserver(p2p *p2p.Server, dbService *database.ShardDB, shardID int) (*Observer, error) {
|
|
ctx, cancel := context.WithCancel(context.Background())
|
|
return &Observer{p2p, dbService, shardID, ctx, cancel}, nil
|
|
}
|
|
|
|
// Start the main loop for observer service.
|
|
func (o *Observer) Start() {
|
|
log.Info(fmt.Sprintf("Starting observer service"))
|
|
// shard := sharding.NewShard(big.NewInt(int64(o.shardID)), o.dbService.DB())
|
|
}
|
|
|
|
// Stop the main loop for observer service.
|
|
func (o *Observer) Stop() error {
|
|
// Triggers a cancel call in the service's context which shuts down every goroutine
|
|
// in this service.
|
|
defer o.cancel()
|
|
log.Info(fmt.Sprintf("Stopping observer service"))
|
|
return nil
|
|
}
|