mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-26 21:27:19 +00:00
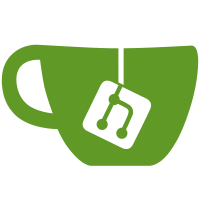
Former-commit-id: b7b8bbd10777012ae6f7d30eb6b05c3b1c3ec5d3 [formerly 06e1112fa0e1092a7137186d3a386972daa2effe] Former-commit-id: ff2bc760c9dafb6250f056606eb2cbf96b6afa5b
69 lines
2.4 KiB
Go
69 lines
2.4 KiB
Go
package mainchain
|
|
|
|
import (
|
|
"context"
|
|
"math/big"
|
|
"time"
|
|
|
|
ethereum "github.com/ethereum/go-ethereum"
|
|
"github.com/ethereum/go-ethereum/accounts"
|
|
"github.com/ethereum/go-ethereum/accounts/abi/bind"
|
|
"github.com/ethereum/go-ethereum/common"
|
|
gethTypes "github.com/ethereum/go-ethereum/core/types"
|
|
"github.com/prysmaticlabs/prysm/client/contracts"
|
|
)
|
|
|
|
// Signer defines an interface that can read from the Ethereum mainchain as well call
|
|
// read-only methods and functions from the Sharding Manager Contract.
|
|
type Signer interface {
|
|
Sign(hash common.Hash) ([]byte, error)
|
|
}
|
|
|
|
// ContractManager specifies an interface that defines both read/write
|
|
// operations on a contract in the Ethereum mainchain.
|
|
type ContractManager interface {
|
|
ContractCaller
|
|
ContractTransactor
|
|
}
|
|
|
|
// ContractCaller defines an interface that can read from a contract on the
|
|
// Ethereum mainchain as well as call its read-only methods and functions.
|
|
type ContractCaller interface {
|
|
SMCCaller() *contracts.SMCCaller
|
|
GetShardCount() (int64, error)
|
|
}
|
|
|
|
// ContractTransactor defines an interface that can transact with a contract on the
|
|
// Ethereum mainchain as well as call its methods and functions.
|
|
type ContractTransactor interface {
|
|
SMCTransactor() *contracts.SMCTransactor
|
|
CreateTXOpts(value *big.Int) (*bind.TransactOpts, error)
|
|
}
|
|
|
|
// EthClient defines the methods that will be used to perform rpc calls
|
|
// to the main geth node, and be responsible for other user-specific data
|
|
type EthClient interface {
|
|
Account() *accounts.Account
|
|
WaitForTransaction(ctx context.Context, hash common.Hash, durationInSeconds time.Duration) error
|
|
TransactionReceipt(hash common.Hash) (*gethTypes.Receipt, error)
|
|
DepositFlag() bool
|
|
}
|
|
|
|
// Reader defines an interface for a struct that can read mainchain information
|
|
// such as blocks, transactions, receipts, and more. Useful for testing.
|
|
type Reader interface {
|
|
BlockByNumber(ctx context.Context, number *big.Int) (*gethTypes.Block, error)
|
|
SubscribeNewHead(ctx context.Context, ch chan<- *gethTypes.Header) (ethereum.Subscription, error)
|
|
}
|
|
|
|
// RecordFetcher serves as an interface for a struct that can fetch collation information
|
|
// from a sharding manager contract on the Ethereum mainchain.
|
|
type RecordFetcher interface {
|
|
CollationRecords(opts *bind.CallOpts, arg0 *big.Int, arg1 *big.Int) (struct {
|
|
ChunkRoot [32]byte
|
|
Proposer common.Address
|
|
IsElected bool
|
|
Signature [32]byte
|
|
}, error)
|
|
}
|