mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-26 21:27:19 +00:00
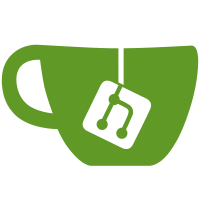
* validator count * fix build errors * fix test error * randao mixes * active indices * lastReceivedMerkleIndex * redundant conversions * eth1FollowDistance * refs * fix compile error * slasher * validator * revert changes to lastReceivedMerkleIndex * remove todo * fix references test * fix committee test * Revert "validator count" This reverts commit 19b376e39914b88b620a113215daa539e3e66758. # Conflicts: # beacon-chain/rpc/beacon/validators.go * Revert "fix build errors" This reverts commit f4acd6e9776c899a0523df10b64e55783b44c451. * Revert "fix test error" This reverts commit 2a5c9eec63e4546139220c71fe3c07ad92bed3b6. * Revert "randao mixes" This reverts commit 2863f9c24dd8cfc44ffce16321f68ef3b793e9a2. * Revert "active indices" This reverts commit 6e8385f3956a08ef9c8980b5343365a1bee48542. * Revert "refs" This reverts commit c64a153f67cd26daa58c3bf5f911da05d41cfb5d. * Revert "fix references test" This reverts commit fe773b55a6f8cc38c6c5c1f96615fdb8bbd4397d. * Revert "fix committee test" This reverts commit 7a0931c4487707e3eaf75a592415577f04253772. * fix compilation errors * Revert "slasher" This reverts commit 8b34137931cff1e6cdffeaf65e1ef07eefdea647. * trieutil * some int() simplifications * fix deepsource issues * removed redundant fmt.Sprint Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com>
135 lines
3.9 KiB
Go
135 lines
3.9 KiB
Go
package cache
|
|
|
|
import (
|
|
"sync"
|
|
"time"
|
|
|
|
lru "github.com/hashicorp/golang-lru"
|
|
"github.com/patrickmn/go-cache"
|
|
"github.com/prysmaticlabs/prysm/shared/params"
|
|
"github.com/prysmaticlabs/prysm/shared/sliceutil"
|
|
)
|
|
|
|
type subnetIDs struct {
|
|
attester *lru.Cache
|
|
attesterLock sync.RWMutex
|
|
aggregator *lru.Cache
|
|
aggregatorLock sync.RWMutex
|
|
persistentSubnets *cache.Cache
|
|
subnetsLock sync.RWMutex
|
|
}
|
|
|
|
// SubnetIDs for attester and aggregator.
|
|
var SubnetIDs = newSubnetIDs()
|
|
|
|
func newSubnetIDs() *subnetIDs {
|
|
// Given a node can calculate committee assignments of current epoch and next epoch.
|
|
// Max size is set to 2 epoch length.
|
|
cacheSize := int(params.BeaconConfig().MaxCommitteesPerSlot * params.BeaconConfig().SlotsPerEpoch * 2)
|
|
attesterCache, err := lru.New(cacheSize)
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
aggregatorCache, err := lru.New(cacheSize)
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
epochDuration := time.Duration(params.BeaconConfig().SlotsPerEpoch * params.BeaconConfig().SecondsPerSlot)
|
|
subLength := epochDuration * time.Duration(params.BeaconNetworkConfig().EpochsPerRandomSubnetSubscription)
|
|
persistentCache := cache.New(subLength*time.Second, epochDuration*time.Second)
|
|
return &subnetIDs{attester: attesterCache, aggregator: aggregatorCache, persistentSubnets: persistentCache}
|
|
}
|
|
|
|
// AddAttesterSubnetID adds the subnet index for subscribing subnet for the attester of a given slot.
|
|
func (c *subnetIDs) AddAttesterSubnetID(slot, subnetID uint64) {
|
|
c.attesterLock.Lock()
|
|
defer c.attesterLock.Unlock()
|
|
|
|
ids := []uint64{subnetID}
|
|
val, exists := c.attester.Get(slot)
|
|
if exists {
|
|
ids = sliceutil.UnionUint64(append(val.([]uint64), ids...))
|
|
}
|
|
c.attester.Add(slot, ids)
|
|
}
|
|
|
|
// GetAttesterSubnetIDs gets the subnet IDs for subscribed subnets for attesters of the slot.
|
|
func (c *subnetIDs) GetAttesterSubnetIDs(slot uint64) []uint64 {
|
|
c.attesterLock.RLock()
|
|
defer c.attesterLock.RUnlock()
|
|
|
|
val, exists := c.attester.Get(slot)
|
|
if !exists {
|
|
return nil
|
|
}
|
|
if v, ok := val.([]uint64); ok {
|
|
return v
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// AddAggregatorSubnetID adds the subnet ID for subscribing subnet for the aggregator of a given slot.
|
|
func (c *subnetIDs) AddAggregatorSubnetID(slot, subnetID uint64) {
|
|
c.aggregatorLock.Lock()
|
|
defer c.aggregatorLock.Unlock()
|
|
|
|
ids := []uint64{subnetID}
|
|
val, exists := c.aggregator.Get(slot)
|
|
if exists {
|
|
ids = sliceutil.UnionUint64(append(val.([]uint64), ids...))
|
|
}
|
|
c.aggregator.Add(slot, ids)
|
|
}
|
|
|
|
// GetAggregatorSubnetIDs gets the subnet IDs for subscribing subnet for aggregator of the slot.
|
|
func (c *subnetIDs) GetAggregatorSubnetIDs(slot uint64) []uint64 {
|
|
c.aggregatorLock.RLock()
|
|
defer c.aggregatorLock.RUnlock()
|
|
|
|
val, exists := c.aggregator.Get(slot)
|
|
if !exists {
|
|
return []uint64{}
|
|
}
|
|
return val.([]uint64)
|
|
}
|
|
|
|
// GetPersistentSubnets retrieves the persistent subnet and expiration time of that validator's
|
|
// subscription.
|
|
func (c *subnetIDs) GetPersistentSubnets(pubkey []byte) ([]uint64, bool, time.Time) {
|
|
c.subnetsLock.RLock()
|
|
defer c.subnetsLock.RUnlock()
|
|
|
|
id, duration, ok := c.persistentSubnets.GetWithExpiration(string(pubkey))
|
|
if !ok {
|
|
return []uint64{}, ok, time.Time{}
|
|
}
|
|
return id.([]uint64), ok, duration
|
|
}
|
|
|
|
// GetAllSubnets retrieves all the non-expired subscribed subnets of all the validators
|
|
// in the cache.
|
|
func (c *subnetIDs) GetAllSubnets() []uint64 {
|
|
c.subnetsLock.RLock()
|
|
defer c.subnetsLock.RUnlock()
|
|
|
|
itemsMap := c.persistentSubnets.Items()
|
|
var committees []uint64
|
|
|
|
for _, v := range itemsMap {
|
|
if v.Expired() {
|
|
continue
|
|
}
|
|
committees = append(committees, v.Object.([]uint64)...)
|
|
}
|
|
return sliceutil.SetUint64(committees)
|
|
}
|
|
|
|
// AddPersistentCommittee adds the relevant committee for that particular validator along with its
|
|
// expiration period.
|
|
func (c *subnetIDs) AddPersistentCommittee(pubkey []byte, comIndex []uint64, duration time.Duration) {
|
|
c.subnetsLock.Lock()
|
|
defer c.subnetsLock.Unlock()
|
|
|
|
c.persistentSubnets.Set(string(pubkey), comIndex, duration)
|
|
}
|