mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-26 05:17:22 +00:00
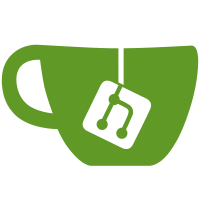
* WIP - slasher highest attestation start * fixed previous * highest source and target * highest attestation cache * cleanup * persist + fixes * PR fixes and cleanup * slashing proto * highest att. api * cleanup + tests * increased highest att. cache to 300K * removed highest att. api (for a separate PR) * fixed linting * bazel build fix * highest att. kv test * slasher highest att. test + purge + fix on eviction persist performance * cleanup + linting * linting + test fixes * bazel gazelle run * PR fixes * run goimports * go mod tidy * ineffectual assignment fix * run gazelle * bazel gazelle run * test fixes * linter fix * Apply suggestions from code review Co-authored-by: Shay Zluf <thezluf@gmail.com> * goimports run * cache tests * A bunch of small fixes * gazelle fix + gofmt * merge fixes * kv ordering fix * small typos and text fixes * capital letter fix Co-authored-by: Shay Zluf <thezluf@gmail.com>
111 lines
2.7 KiB
Go
111 lines
2.7 KiB
Go
package cache
|
|
|
|
import (
|
|
"testing"
|
|
|
|
ethereum_slashing "github.com/prysmaticlabs/prysm/proto/slashing"
|
|
|
|
"github.com/prysmaticlabs/prysm/shared/testutil/require"
|
|
)
|
|
|
|
func TestStoringAndFetching(t *testing.T) {
|
|
cache, err := NewHighestAttestationCache(10, nil)
|
|
require.NoError(t, err)
|
|
|
|
// Cache a test attestation.
|
|
cache.Set(1, ðereum_slashing.HighestAttestation{
|
|
ValidatorId: 1,
|
|
HighestSourceEpoch: 2,
|
|
HighestTargetEpoch: 3,
|
|
})
|
|
|
|
// Require it to exist.
|
|
require.Equal(t, true, cache.Has(1))
|
|
|
|
// fetch
|
|
res, b := cache.Get(1)
|
|
require.Equal(t, true, b)
|
|
require.Equal(t, uint64(1), res[1].ValidatorId)
|
|
require.Equal(t, uint64(2), res[1].HighestSourceEpoch)
|
|
require.Equal(t, uint64(3), res[1].HighestTargetEpoch)
|
|
|
|
// Delete it.
|
|
require.Equal(t, true, cache.Delete(1))
|
|
// Confirm deletion.
|
|
res2, b2 := cache.Get(1)
|
|
require.Equal(t, false, b2)
|
|
require.Equal(t, true, res2 == nil)
|
|
}
|
|
|
|
func TestPurge(t *testing.T) {
|
|
wasEvicted := false
|
|
onEvicted := func(key interface{}, value interface{}) {
|
|
wasEvicted = true
|
|
}
|
|
cache, err := NewHighestAttestationCache(10, onEvicted)
|
|
require.NoError(t, err)
|
|
|
|
// Cache several test attestation.
|
|
cache.Set(1, ðereum_slashing.HighestAttestation{
|
|
ValidatorId: 1,
|
|
HighestSourceEpoch: 2,
|
|
HighestTargetEpoch: 3,
|
|
})
|
|
cache.Set(2, ðereum_slashing.HighestAttestation{
|
|
ValidatorId: 4,
|
|
HighestSourceEpoch: 5,
|
|
HighestTargetEpoch: 6,
|
|
})
|
|
cache.Set(3, ðereum_slashing.HighestAttestation{
|
|
ValidatorId: 7,
|
|
HighestSourceEpoch: 8,
|
|
HighestTargetEpoch: 9,
|
|
})
|
|
|
|
cache.Purge()
|
|
|
|
// Require all attestations to be deleted
|
|
require.Equal(t, false, cache.Has(1))
|
|
require.Equal(t, false, cache.Has(2))
|
|
require.Equal(t, false, cache.Has(3))
|
|
|
|
// Require the eviction function to be called.
|
|
require.Equal(t, true, wasEvicted)
|
|
}
|
|
|
|
func TestClear(t *testing.T) {
|
|
wasEvicted := false
|
|
onEvicted := func(key interface{}, value interface{}) {
|
|
wasEvicted = true
|
|
}
|
|
cache, err := NewHighestAttestationCache(10, onEvicted)
|
|
require.NoError(t, err)
|
|
|
|
// Cache several test attestation.
|
|
cache.Set(1, ðereum_slashing.HighestAttestation{
|
|
ValidatorId: 1,
|
|
HighestSourceEpoch: 2,
|
|
HighestTargetEpoch: 3,
|
|
})
|
|
cache.Set(2, ðereum_slashing.HighestAttestation{
|
|
ValidatorId: 4,
|
|
HighestSourceEpoch: 5,
|
|
HighestTargetEpoch: 6,
|
|
})
|
|
cache.Set(3, ðereum_slashing.HighestAttestation{
|
|
ValidatorId: 7,
|
|
HighestSourceEpoch: 8,
|
|
HighestTargetEpoch: 9,
|
|
})
|
|
|
|
cache.Clear()
|
|
|
|
// Require all attestations to be deleted
|
|
require.Equal(t, false, cache.Has(1))
|
|
require.Equal(t, false, cache.Has(2))
|
|
require.Equal(t, false, cache.Has(3))
|
|
|
|
// Require the eviction function to be called.
|
|
require.Equal(t, true, wasEvicted)
|
|
}
|