mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-22 19:40:37 +00:00
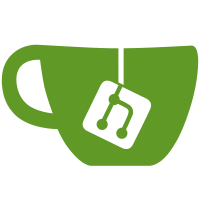
* Update V3 from V4 * Fix build v3 -> v4 * Update ssz * Update beacon_chain.pb.go * Fix formatter import * Update update-mockgen.sh comment to v4 * Fix conflicts. Pass build and tests * Fix test
51 lines
1.5 KiB
Go
51 lines
1.5 KiB
Go
package state_native
|
|
|
|
import (
|
|
ethpb "github.com/prysmaticlabs/prysm/v4/proto/prysm/v1alpha1"
|
|
"github.com/prysmaticlabs/prysm/v4/runtime/version"
|
|
)
|
|
|
|
// PreviousEpochAttestations corresponding to blocks on the beacon chain.
|
|
func (b *BeaconState) PreviousEpochAttestations() ([]*ethpb.PendingAttestation, error) {
|
|
if b.version != version.Phase0 {
|
|
return nil, errNotSupported("PreviousEpochAttestations", b.version)
|
|
}
|
|
|
|
if b.previousEpochAttestations == nil {
|
|
return nil, nil
|
|
}
|
|
|
|
b.lock.RLock()
|
|
defer b.lock.RUnlock()
|
|
|
|
return b.previousEpochAttestationsVal(), nil
|
|
}
|
|
|
|
// previousEpochAttestationsVal corresponding to blocks on the beacon chain.
|
|
// This assumes that a lock is already held on BeaconState.
|
|
func (b *BeaconState) previousEpochAttestationsVal() []*ethpb.PendingAttestation {
|
|
return ethpb.CopyPendingAttestationSlice(b.previousEpochAttestations)
|
|
}
|
|
|
|
// CurrentEpochAttestations corresponding to blocks on the beacon chain.
|
|
func (b *BeaconState) CurrentEpochAttestations() ([]*ethpb.PendingAttestation, error) {
|
|
if b.version != version.Phase0 {
|
|
return nil, errNotSupported("CurrentEpochAttestations", b.version)
|
|
}
|
|
|
|
if b.currentEpochAttestations == nil {
|
|
return nil, nil
|
|
}
|
|
|
|
b.lock.RLock()
|
|
defer b.lock.RUnlock()
|
|
|
|
return b.currentEpochAttestationsVal(), nil
|
|
}
|
|
|
|
// currentEpochAttestations corresponding to blocks on the beacon chain.
|
|
// This assumes that a lock is already held on BeaconState.
|
|
func (b *BeaconState) currentEpochAttestationsVal() []*ethpb.PendingAttestation {
|
|
return ethpb.CopyPendingAttestationSlice(b.currentEpochAttestations)
|
|
}
|