mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 21:07:18 +00:00
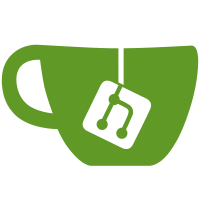
* Remove native state flag and use native state in spectests * remove feature from tests * use e2e config in slasher simulator * use params.BeaconConfig in testutil * use correct function * use minimal config in go_test * fix TestListValidators * parameterize sync committee bits and aggregation bits * Fix TestServer_ListIndexedAttestations_GenesisEpoch (cherry picked from commit 254ab623dde08ae8886b152facdbbd8889ed79db) * fix more tests * fix even more * moreeee * aaaand more * one more fix * one more * simplify TestGetAltairDuties_UnknownPubkey * comment out problematic test * one more fix * one more * aaaand one more * another * use fieldparams in HydrateBlindedBeaconBlockBodyBellatrix * create new package for mainnet tests * TestServer_GetBellatrixBeaconBlock * change slashed validator index * clear cache in reward_test.go * deprecate flag * create bazel mainnet target * move attester mainnet test to mainnet target * "fix" proposer tests * use minimal config in TestServer_circuitBreakBuilder * fix TestProposer_ProposeBlock_OK * more fixes in validator package * more fixes * more fixes * test code * move TestProposer_GetBeaconBlock_BellatrixEpoch to minimal * finally * remove proposer_bellatrix_mainnet_test.go * fix TestServer_GetBellatrixBeaconBlock_HappyCase * fix TestServer_GetBellatrixBeaconBlock_BuilderCase * Preston needs to fix this! * Revert "Preston needs to fix this!" This reverts commit b03d97a16e3080e254c7b19d7f193d3c600ca869. * remove proto state tests * fix migration tests * static analysis fix * review * remove proto state * swap state in tests * fix BUILD file in /proto/testing * remove metrics test with nil state
303 lines
7.2 KiB
Go
303 lines
7.2 KiB
Go
package helpers
|
|
|
|
import (
|
|
"strconv"
|
|
"testing"
|
|
|
|
state_native "github.com/prysmaticlabs/prysm/v3/beacon-chain/state/state-native"
|
|
"github.com/prysmaticlabs/prysm/v3/config/params"
|
|
types "github.com/prysmaticlabs/prysm/v3/consensus-types/primitives"
|
|
ethpb "github.com/prysmaticlabs/prysm/v3/proto/eth/v1"
|
|
"github.com/prysmaticlabs/prysm/v3/proto/migration"
|
|
"github.com/prysmaticlabs/prysm/v3/testing/assert"
|
|
"github.com/prysmaticlabs/prysm/v3/testing/require"
|
|
)
|
|
|
|
func Test_ValidatorStatus(t *testing.T) {
|
|
farFutureEpoch := params.BeaconConfig().FarFutureEpoch
|
|
|
|
type args struct {
|
|
validator *ethpb.Validator
|
|
epoch types.Epoch
|
|
}
|
|
tests := []struct {
|
|
name string
|
|
args args
|
|
want ethpb.ValidatorStatus
|
|
wantErr bool
|
|
}{
|
|
{
|
|
name: "pending initialized",
|
|
args: args{
|
|
validator: ðpb.Validator{
|
|
ActivationEpoch: farFutureEpoch,
|
|
ActivationEligibilityEpoch: farFutureEpoch,
|
|
},
|
|
epoch: types.Epoch(5),
|
|
},
|
|
want: ethpb.ValidatorStatus_PENDING,
|
|
},
|
|
{
|
|
name: "pending queued",
|
|
args: args{
|
|
validator: ðpb.Validator{
|
|
ActivationEpoch: 10,
|
|
ActivationEligibilityEpoch: 2,
|
|
},
|
|
epoch: types.Epoch(5),
|
|
},
|
|
want: ethpb.ValidatorStatus_PENDING,
|
|
},
|
|
{
|
|
name: "active ongoing",
|
|
args: args{
|
|
validator: ðpb.Validator{
|
|
ActivationEpoch: 3,
|
|
ExitEpoch: farFutureEpoch,
|
|
},
|
|
epoch: types.Epoch(5),
|
|
},
|
|
want: ethpb.ValidatorStatus_ACTIVE,
|
|
},
|
|
{
|
|
name: "active slashed",
|
|
args: args{
|
|
validator: ðpb.Validator{
|
|
ActivationEpoch: 3,
|
|
ExitEpoch: 30,
|
|
Slashed: true,
|
|
},
|
|
epoch: types.Epoch(5),
|
|
},
|
|
want: ethpb.ValidatorStatus_ACTIVE,
|
|
},
|
|
{
|
|
name: "active exiting",
|
|
args: args{
|
|
validator: ðpb.Validator{
|
|
ActivationEpoch: 3,
|
|
ExitEpoch: 30,
|
|
Slashed: false,
|
|
},
|
|
epoch: types.Epoch(5),
|
|
},
|
|
want: ethpb.ValidatorStatus_ACTIVE,
|
|
},
|
|
{
|
|
name: "exited slashed",
|
|
args: args{
|
|
validator: ðpb.Validator{
|
|
ActivationEpoch: 3,
|
|
ExitEpoch: 30,
|
|
WithdrawableEpoch: 40,
|
|
Slashed: true,
|
|
},
|
|
epoch: types.Epoch(35),
|
|
},
|
|
want: ethpb.ValidatorStatus_EXITED,
|
|
},
|
|
{
|
|
name: "exited unslashed",
|
|
args: args{
|
|
validator: ðpb.Validator{
|
|
ActivationEpoch: 3,
|
|
ExitEpoch: 30,
|
|
WithdrawableEpoch: 40,
|
|
Slashed: false,
|
|
},
|
|
epoch: types.Epoch(35),
|
|
},
|
|
want: ethpb.ValidatorStatus_EXITED,
|
|
},
|
|
{
|
|
name: "withdrawal possible",
|
|
args: args{
|
|
validator: ðpb.Validator{
|
|
ActivationEpoch: 3,
|
|
ExitEpoch: 30,
|
|
WithdrawableEpoch: 40,
|
|
EffectiveBalance: params.BeaconConfig().MaxEffectiveBalance,
|
|
Slashed: false,
|
|
},
|
|
epoch: types.Epoch(45),
|
|
},
|
|
want: ethpb.ValidatorStatus_WITHDRAWAL,
|
|
},
|
|
{
|
|
name: "withdrawal done",
|
|
args: args{
|
|
validator: ðpb.Validator{
|
|
ActivationEpoch: 3,
|
|
ExitEpoch: 30,
|
|
WithdrawableEpoch: 40,
|
|
EffectiveBalance: 0,
|
|
Slashed: false,
|
|
},
|
|
epoch: types.Epoch(45),
|
|
},
|
|
want: ethpb.ValidatorStatus_WITHDRAWAL,
|
|
},
|
|
}
|
|
for _, tt := range tests {
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
readOnlyVal, err := state_native.NewValidator(migration.V1ValidatorToV1Alpha1(tt.args.validator))
|
|
require.NoError(t, err)
|
|
got, err := ValidatorStatus(readOnlyVal, tt.args.epoch)
|
|
require.NoError(t, err)
|
|
if got != tt.want {
|
|
t.Errorf("validatorStatus() got = %v, want %v", got, tt.want)
|
|
}
|
|
})
|
|
}
|
|
}
|
|
|
|
func Test_ValidatorSubStatus(t *testing.T) {
|
|
farFutureEpoch := params.BeaconConfig().FarFutureEpoch
|
|
|
|
type args struct {
|
|
validator *ethpb.Validator
|
|
epoch types.Epoch
|
|
}
|
|
tests := []struct {
|
|
name string
|
|
args args
|
|
want ethpb.ValidatorStatus
|
|
wantErr bool
|
|
}{
|
|
{
|
|
name: "pending initialized",
|
|
args: args{
|
|
validator: ðpb.Validator{
|
|
ActivationEpoch: farFutureEpoch,
|
|
ActivationEligibilityEpoch: farFutureEpoch,
|
|
},
|
|
epoch: types.Epoch(5),
|
|
},
|
|
want: ethpb.ValidatorStatus_PENDING_INITIALIZED,
|
|
},
|
|
{
|
|
name: "pending queued",
|
|
args: args{
|
|
validator: ðpb.Validator{
|
|
ActivationEpoch: 10,
|
|
ActivationEligibilityEpoch: 2,
|
|
},
|
|
epoch: types.Epoch(5),
|
|
},
|
|
want: ethpb.ValidatorStatus_PENDING_QUEUED,
|
|
},
|
|
{
|
|
name: "active ongoing",
|
|
args: args{
|
|
validator: ðpb.Validator{
|
|
ActivationEpoch: 3,
|
|
ExitEpoch: farFutureEpoch,
|
|
},
|
|
epoch: types.Epoch(5),
|
|
},
|
|
want: ethpb.ValidatorStatus_ACTIVE_ONGOING,
|
|
},
|
|
{
|
|
name: "active slashed",
|
|
args: args{
|
|
validator: ðpb.Validator{
|
|
ActivationEpoch: 3,
|
|
ExitEpoch: 30,
|
|
Slashed: true,
|
|
},
|
|
epoch: types.Epoch(5),
|
|
},
|
|
want: ethpb.ValidatorStatus_ACTIVE_SLASHED,
|
|
},
|
|
{
|
|
name: "active exiting",
|
|
args: args{
|
|
validator: ðpb.Validator{
|
|
ActivationEpoch: 3,
|
|
ExitEpoch: 30,
|
|
Slashed: false,
|
|
},
|
|
epoch: types.Epoch(5),
|
|
},
|
|
want: ethpb.ValidatorStatus_ACTIVE_EXITING,
|
|
},
|
|
{
|
|
name: "exited slashed",
|
|
args: args{
|
|
validator: ðpb.Validator{
|
|
ActivationEpoch: 3,
|
|
ExitEpoch: 30,
|
|
WithdrawableEpoch: 40,
|
|
Slashed: true,
|
|
},
|
|
epoch: types.Epoch(35),
|
|
},
|
|
want: ethpb.ValidatorStatus_EXITED_SLASHED,
|
|
},
|
|
{
|
|
name: "exited unslashed",
|
|
args: args{
|
|
validator: ðpb.Validator{
|
|
ActivationEpoch: 3,
|
|
ExitEpoch: 30,
|
|
WithdrawableEpoch: 40,
|
|
Slashed: false,
|
|
},
|
|
epoch: types.Epoch(35),
|
|
},
|
|
want: ethpb.ValidatorStatus_EXITED_UNSLASHED,
|
|
},
|
|
{
|
|
name: "withdrawal possible",
|
|
args: args{
|
|
validator: ðpb.Validator{
|
|
ActivationEpoch: 3,
|
|
ExitEpoch: 30,
|
|
WithdrawableEpoch: 40,
|
|
EffectiveBalance: params.BeaconConfig().MaxEffectiveBalance,
|
|
Slashed: false,
|
|
},
|
|
epoch: types.Epoch(45),
|
|
},
|
|
want: ethpb.ValidatorStatus_WITHDRAWAL_POSSIBLE,
|
|
},
|
|
{
|
|
name: "withdrawal done",
|
|
args: args{
|
|
validator: ðpb.Validator{
|
|
ActivationEpoch: 3,
|
|
ExitEpoch: 30,
|
|
WithdrawableEpoch: 40,
|
|
EffectiveBalance: 0,
|
|
Slashed: false,
|
|
},
|
|
epoch: types.Epoch(45),
|
|
},
|
|
want: ethpb.ValidatorStatus_WITHDRAWAL_DONE,
|
|
},
|
|
}
|
|
for _, tt := range tests {
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
readOnlyVal, err := state_native.NewValidator(migration.V1ValidatorToV1Alpha1(tt.args.validator))
|
|
require.NoError(t, err)
|
|
got, err := ValidatorSubStatus(readOnlyVal, tt.args.epoch)
|
|
require.NoError(t, err)
|
|
if got != tt.want {
|
|
t.Errorf("validatorSubStatus() got = %v, want %v", got, tt.want)
|
|
}
|
|
})
|
|
}
|
|
}
|
|
|
|
// This test verifies how many validator statuses have meaningful values.
|
|
// The first expected non-meaningful value will have x.String() equal to its numeric representation.
|
|
// This test assumes we start numbering from 0 and do not skip any values.
|
|
// Having a test like this allows us to use e.g. `if value < 10` for validity checks.
|
|
func TestNumberOfStatuses(t *testing.T) {
|
|
lastValidEnumValue := 12
|
|
x := ethpb.ValidatorStatus(lastValidEnumValue)
|
|
assert.NotEqual(t, strconv.Itoa(lastValidEnumValue), x.String())
|
|
x = ethpb.ValidatorStatus(lastValidEnumValue + 1)
|
|
assert.Equal(t, strconv.Itoa(lastValidEnumValue+1), x.String())
|
|
}
|