mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-25 12:57:18 +00:00
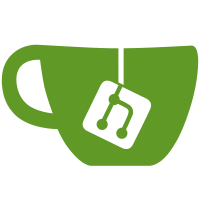
* add new method * Merge branch 'master' into addMapHandler * comments * Merge branch 'addMapHandler' of https://github.com/prysmaticlabs/geth-sharding into addMapHandler * Update beacon-chain/state/types.go Co-authored-by: terence tsao <terence@prysmaticlabs.com> * Merge refs/heads/master into addMapHandler * Merge refs/heads/master into addMapHandler * Merge refs/heads/master into addMapHandler * Merge refs/heads/master into addMapHandler * Merge refs/heads/master into addMapHandler * Merge refs/heads/master into addMapHandler * Merge refs/heads/master into addMapHandler * Merge refs/heads/master into addMapHandler * gofmt
38 lines
1.3 KiB
Go
38 lines
1.3 KiB
Go
package state
|
|
|
|
import (
|
|
"strconv"
|
|
"testing"
|
|
|
|
ethpb "github.com/prysmaticlabs/ethereumapis/eth/v1alpha1"
|
|
"github.com/prysmaticlabs/prysm/shared/bytesutil"
|
|
"github.com/prysmaticlabs/prysm/shared/params"
|
|
"github.com/prysmaticlabs/prysm/shared/testutil/assert"
|
|
)
|
|
|
|
func TestValidatorMap_DistinctCopy(t *testing.T) {
|
|
count := uint64(100)
|
|
vals := make([]*ethpb.Validator, 0, count)
|
|
for i := uint64(1); i < count; i++ {
|
|
someRoot := [32]byte{}
|
|
someKey := [48]byte{}
|
|
copy(someRoot[:], strconv.Itoa(int(i)))
|
|
copy(someKey[:], strconv.Itoa(int(i)))
|
|
vals = append(vals, ðpb.Validator{
|
|
PublicKey: someKey[:],
|
|
WithdrawalCredentials: someRoot[:],
|
|
EffectiveBalance: params.BeaconConfig().MaxEffectiveBalance,
|
|
Slashed: false,
|
|
ActivationEligibilityEpoch: 1,
|
|
ActivationEpoch: 1,
|
|
ExitEpoch: 1,
|
|
WithdrawableEpoch: 1,
|
|
})
|
|
}
|
|
handler := newValHandler(vals)
|
|
newHandler := handler.copy()
|
|
wantedPubkey := strconv.Itoa(22)
|
|
handler.valIdxMap[bytesutil.ToBytes48([]byte(wantedPubkey))] = 27
|
|
assert.NotEqual(t, handler.valIdxMap[bytesutil.ToBytes48([]byte(wantedPubkey))], newHandler.valIdxMap[bytesutil.ToBytes48([]byte(wantedPubkey))], "Values are supposed to be unequal due to copy")
|
|
}
|