mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-26 05:17:22 +00:00
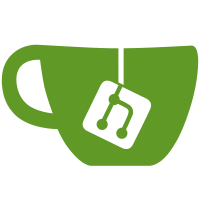
* init-sync updates * slasher/db/kv tests * beacon-chain/rpc/beacon tests * update kv_test * beacon-chain/rpc-validator tests updated * slasher/db/kv - remove teardown method * beacon-chain/sync tests updated * beacon-chain/db/kv tests updated * beacon-chain/blockchain tests updated * beacon-chain/state/stategen tests updated * beacon-chain/powchain updates * updates rest of slasher tests * validator/db tests * rest of the tests * minor comments update * gazelle * Merge refs/heads/master into teardowndb-to-cleanup
47 lines
1.1 KiB
Go
47 lines
1.1 KiB
Go
package db
|
|
|
|
import (
|
|
"crypto/rand"
|
|
"fmt"
|
|
"math/big"
|
|
"os"
|
|
"path/filepath"
|
|
"testing"
|
|
)
|
|
|
|
// SetupDB instantiates and returns a DB instance for the validator client.
|
|
func SetupDB(t testing.TB, pubkeys [][48]byte) *Store {
|
|
randPath, err := rand.Int(rand.Reader, big.NewInt(1000000))
|
|
if err != nil {
|
|
t.Fatalf("Could not generate random file path: %v", err)
|
|
}
|
|
p := filepath.Join(TempDir(), fmt.Sprintf("/%d", randPath))
|
|
if err := os.RemoveAll(p); err != nil {
|
|
t.Fatalf("Failed to remove directory: %v", err)
|
|
}
|
|
db, err := NewKVStore(p, pubkeys)
|
|
if err != nil {
|
|
t.Fatalf("Failed to instantiate DB: %v", err)
|
|
}
|
|
t.Cleanup(func() {
|
|
if err := db.Close(); err != nil {
|
|
t.Fatalf("Failed to close database: %v", err)
|
|
}
|
|
if err := db.ClearDB(); err != nil {
|
|
t.Fatalf("Failed to clear database: %v", err)
|
|
}
|
|
})
|
|
return db
|
|
}
|
|
|
|
// TempDir returns a directory path for temporary test storage.
|
|
func TempDir() string {
|
|
d := os.Getenv("TEST_TMPDIR")
|
|
|
|
// If the test is not run via bazel, the environment var won't be set.
|
|
if d == "" {
|
|
return os.TempDir()
|
|
}
|
|
return d
|
|
}
|